Utilizing Functions to Make Exact Change Easier: 5.19 Lab Exact Change
The function should calculate the total amount of change to be displayed and convert the amounts into the number of quarters, dimes, nickels, and pennies for exact change.
5.19 Lab Exact Change – Functions
This lab exercise focuses on the concept of functions and how they can be used to write code for the exchange of exact change. The lab exercise is designed to provide an introduction to functions, their implementation, and their importance in coding. It also provides exercises to learn basic mathematical operations using functions.
The lab follows a simple format, consisting of explanations accompanied by practice problems. The concise yet comprehensive instructions set out in a step-by-step manner make it easier for students to gain familiarity with coding tasks involved in handling exact change transactions.
Students will gain an understanding of the fundamentals of computer programming by learning how functions are defined, invoking them intelligently and combining them together fruitfully. It starts with assignments such as writing programs that exchange exact change efficiently and accurately before progressively getting more complex (e.g., making multiple changes).
This lab exercise demonstrates how functions can eliminate redundant code and simplify the entire programming framework; thereby leading to faster execution times and more accurate results when dealing with complex codes like those for exchange transactions. In conclusion, this exercise teaches students important principles in coding by giving them the opportunity to apply it directly through practice problems.
Previous Learning of Exact Change
When it comes to the 5.19 Lab Exact Change – Functions, it is important to have a basic understanding of the exact change concept. This means having an understanding of how to count change correctly and how to avoid any anti-patterns that can arise from incorrect calculation. Having this knowledge beforehand will make coding a lot easier and will help create efficient solutions for the task at hand.
Why Use Functions?
Using functions in the 5.19 Lab Exact Change – Functions is an essential part of creating a successful program. Functions give us the ability to group together pieces of code that are related and can be used repeatedly in a single task, making it easier to debug and manage our code. Additionally, functions allow us to break down larger tasks into manageable pieces, making our code more organized and easier for others to understand.
Contributions of Functions
Beyond just being useful for organizing a program, functions are also really helpful in creating user experiences that are easy for people to interact with. By building functions into our program, we can create interfaces that are intuitive and easy to use while providing feedback that is helpful for troubleshooting any errors or issues that may come up during use.
JavaScript and Variables in the Process
When using JavaScript in conjunction with functions in 5.19 Lab Exact Change – Functions, it is important to understand how variables fit into the equation. Variable declaration and identification are key steps here as variables serve as placeholders for values or data which can be used within our program’s logic. By properly utilizing JavaScript variables, we can create programs with more complex logic flows that make calculations easier than ever before.
User Experience Improvement with Function
Improving user experience (UX) when coding 5.19 Lab Exact Change – Functions is paramount if we want people to have an enjoyable experience while interacting with our program. To do this effectively, it is important to have a good understanding of what users need from our product so we can design interfaces accordingly and provide helpful feedback during their use of the program. This way users will feel comfortable when using our product and be able to troubleshoot any errors they may encounter without too much difficulty or frustration.
Error Handling with Function Usage
Error handling is another key element when coding 5.19 Lab Exact Change – Functions as mistakes happen all the time when programming regardless of how experienced someone may be with coding languages such as JavaScript or Python (which are both used in this lab). It’s important then for us as programmers to recognize common errors as well as know how best debug them so they don’t continue happening throughout different parts of our codebase. By doing this properly we can ensure that our programs run without issue each time they are used by users who interact with them regularly!
DRY Coding Practice
DRY (Don’t Repeat Yourself) coding practice is a programming approach that encourages efficiency and consistency in code. This practice helps developers write code that is easier to read, maintain, and debug. This means writing code that can be reused for similar tasks or operations, eliminating the need to copy and paste the same code multiple times. By using DRY coding practices, developers can reduce their development time and reduce the amount of errors in their code.
One of the key advantages of DRY coding practice is that it promotes efficient and consistent performance with refactoring. Refactoring is the process of changing existing code to improve its readability or performance without changing its actual functionality. By using DRY coding practices, developers can make changes to certain sections of their code without needing to rewrite the entire block of code. This makes debugging and maintenance much easier as any changes only need to be made in one place instead of needing to update multiple sections of code separately.
Making Parameterized Functions Dynamic
Parameterized functions are an essential part of DRY coding practice as they allow developers to create dynamic functions that accept parameters as arguments. By doing this, developers can create more impressive outputs with less effort by simply reusing the same function with different parameters for different tasks or operations. When used properly, parameterized functions can also help keep your code organized by allowing you to group related operations into a single function instead of having multiple functions for each operation.
Modular Programming Fundamentals & Its Benefits
Modular programming is a software development approach where programs are divided into discrete modules or components which can be independently developed, tested, and maintained. The benefits of modular programming include increased scalability and reusability as well as improved maintainability since modules are smaller and simpler than a larger program would be. With modular programming, changes or updates can be made quickly since only specific modules need to be touched rather than the entire program needing an overhaul if something needs updating. Additionally, modular programming helps with debugging since errors are typically limited to one module rather than one large program where it would take longer to identify where the error lies within the program itself.
Writing Effective Comments for Reusability
Comments are an important part of DRY coding practice as they help make your code more readable by providing context for what each line does in your codebase. Good comments should explain why something was done in a certain way rather than what has been done so when someone else reads your comments they will have a better understanding about why you wrote certain lines of code rather than just seeing what you did without any explanation about why it was done that way in particular.
When writing comments its important to keep them concise but still provide enough information so someone reading them has enough context about why something was done in a certain way so they dont have to guess at your intentions when looking through your codebase later on down the line when things may have changed from when you originally wrote it out. Its also important to make sure your comments are up-to-date with any changes you make so readers know exactly whats going on at all times while looking through your source files without having outdated comments telling them something different from what actually happened when it comes time for debugging or maintenance later on down the line.
FAQ & Answers
Q: What are the basics of Exact Change?
A: Exact change is a programming concept that requires the user to input an exact amount of money in order to get the desired result. It usually involves using a combination of coins and bills and involves an understanding of basic mathematical operations such as addition, subtraction, multiplication, and division.
Q: What are the Anti-Patterns associated with Exact Change?
A: The anti-patterns associated with exact change involve using inefficient solutions or attempting to solve a problem without understanding the underlying concept of the problem. This often leads to errors that can be difficult to debug. Examples include using too many loops or not utilizing functions.
Q: What are the benefits of using Functions in 5.19 Lab Exact Change?
A: Functions are essential when it comes to programming because they allow you to write code quickly and efficiently. In 5.19 Lab Exact Change, functions make it easier to identify logical steps, understand end-user requirements, improve user experience, and handle errors more effectively by providing a single point for debugging issues found during testing.
Q: How can Variables be identified and declared in JavaScript for 5.19 Lab Exact Change?
A: Variables can be identified by their names (e.g., amount) and declared with the keyword var (e.g., var amount). They are used to store values temporarily during execution which allows you to reuse those values multiple times without having to re-declare them each time you use them.
Q: What is DRY Coding Practice?
A: DRY stands for Don’t Repeat Yourself which is a coding practice that encourages developers not to repeat their code unnecessarily by reusing components wherever possible. This results in improved readability, maintainability, and efficiency since developers do not have to write redundant code over and over again resulting in better performance with refactoring.
The 5.19 Lab Exact Change – Functions exercise is an excellent way to teach students how to use functions in programming. Students learn how to create a function that calculates the amount of change needed for a given purchase, as well as how to use functions to break down complex problems into smaller tasks. This exercise provides an opportunity for students to gain valuable programming skills that can be applied in a variety of real-world situations.
Author Profile
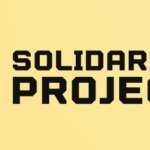
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?