7.6.9 Part 1: Removing All Characters From a String The Ultimate Guide
To remove all characters from a string, use the replace() method to set all the characters to an empty string.
7.6.9 Part 1 Remove All From String
7.6.9 Part 1 Remove All From String is a powerful and easy-to-use utility to help streamline the text processing process. It provides the capability to remove all unwanted content from a string, allowing users to quickly reduce character or word counts, clean up messy text, and simplify the automation of complex text tasks. Its robust algorithm guarantees precise results while working with any type of input character set, such as UTF-8, Unicode, or textual data from HTML encoding. Furthermore, its innovative “Perplexity” mode can detect pesky double words and automatically eliminate them without any manual effort. Finally, its Burstiness mode helps to quickly break long sentences down into smaller more manageable pieces for further processing, saving both time and effort.
7.6.9 Part 1 Remove All From String
Removing all characters, whitespaces, numbers, vowels or a specific character from a string can be easily achieved using the JavaScript method .prototype.replace() and regular expressions. This method allows developers to search for a string within another string and replace it with a new one.
Remove All Characters From String
The .prototype.replace() method is used to remove all characters from a string by searching for them and replacing them with an empty value of newline or no value at all. The syntax used to achieve this is as follows: string.prototype.replace(/[characters_to_be_removed]/g,””) where the characters_to_be_removed are the characters that you want to remove from the string. For example, if you wanted to remove all alphabetic characters from a string, you would use:
string.prototype.replace(/[a-zA-Z]/g,””).
Alternatively, you can use regular expressions to achieve the same result by searching for any character that matches the regular expression /[a-zA-Z]/g and replacing it with an empty value of newline or no value at all – just like in the example above – string.prototype.replace(/[a-zA-Z]/g,””).
Remove All Whitespaces From String
The .prototype.replace() method can also be used to remove all whitespaces from a string by searching for them and replacing them with an empty value of newline or no value at all. The syntax used to achieve this is as follows:
string.prototype.replace(/\s+/g,””) where \s+ is used to represent any whitespace character (including tab spaces). Alternatively, you can use regular expressions to achieve the same result by searching for any character that matches the regular expression /\s+/g and replacing it with an empty value of newline or no value at all – just like in the example above -string.prototype.replace(/\s+/g,””).
Remove All Numbers From String
Removing all numbers from a string can also be accomplished using .prototype.replace() and regular expressions by searching for any character that matches the regular expression /[0-9]/g and replacing it with an empty value of newline or no value at all – just like in the example below -string.prototype.replace(/[0-9]/g,””).
Remove All Vowels From String
Vowels can be removed from strings using either .prototype replace() or regular expressions as well, by searching for any character that matches either [aeiouAEIOU] (for lowercase and uppercase vowels) or [aeiouyAEIOUY] (for lowercase and uppercase vowels including y) and replacing it with an empty value of newline or no value at all – just like in these examples -string1 = string1).prototype replace([aeiouAEIOU], “”); //remove only lowercase & uppercase vowels OR string1 = string1).prototype replace([aeiouyAEIOUY], “”); //remove lowercase & uppercase vowels including y
Remove Specific Character From String
Specific characters can also be removed from strings using either .prototype replace() or regular expressions by searching for any character that matches either [character_to_be_removed] (for exact matching) or [^character_to_be_removed] (for inverse matching) where “character_to_be_removed” represents whichever character you want removed from your original string, followed by an empty space (or nothing), followed by “global” flag (“g”) which tells JavaScript to search through entire text instead of stopping after first occurrence found; such as: Example 1:string1 = string1).prototype replace([character], “”); //remove only one specific character Example 2:string1 = string1).prototype replace([^character], “”); //remove everything except one specific character You should note however that if there are multiple occurrences of said specific character within your original text then only first occurrence will be replaced when using Example 1 whereas multiple occurrences will be replaced when using Example 2 due its inverse matching criteria
Convert To Lowercase And Uppercase String
The easiest way to convert a string to either lowercase or uppercase is to use the JavaScript built-in methods .toLowerCase() and .toUpperCase(). These methods are part of the String.prototype object and are used to convert all of the characters in a string into either lowercase or uppercase. For example, if you have a string “Hello World” and you want to convert it to all lowercase letters, you can do so by using the following code snippet:
let myString = "Hello World";
let myLowerCase = myString.toLowerCase(); // hello world
Similarly, if you want to convert this string into all uppercase letters, you can use the following code snippet:
let myUpperCase = myString.toUpperCase(); // HELLO WORLD
This is a great way to quickly convert strings without having to write custom functions or use external libraries.
Trimming The Start and End of The String
Another useful method available on the String.prototype object is .trim(). This method allows us to trim off any whitespace from the start and end of a string. This is especially useful when dealing with user input as it ensures that no extra whitespace is included in our strings. To trim a given string, we can simply call .trim() on our string like so:
let myString = " Hello World ";
let trimmedString = myString.trim(); // Hello World
In addition to this built-in method, we can also create custom functions for trimming strings if we need more sophisticated trimming functionality than what is provided by .trim(). For example, if we want to only remove whitespace from the beginning of our strings, we could create a custom function like so:
function trimStart(str) {
let trimmedStr = "";
for (let i = 0; i < str.length; i++) { if (str[i] !== ' ') { // If current character isn't whitespace trimmedStr += str[i]; } } return trimmedStr; }
Now that we have our custom function, we can easily trim off any leading whitespace from our strings: let myTrimmedString = trimStart(" Hello World"); // Hello World
.
Repeat The Characters Of Given String
We can repeat characters in a given string using the JavaScript built-in method .repeat(). This method takes an integer argument which represents how many times the characters should be repeated and returns a new string that contains those repeated characters. For example, if we wanted to repeat the word "Hello" 5 times, we could do so using the following code snippet: let myRepeatedString = "Hello".repeat(5); // HelloHelloHelloHelloHello
. In addition to using this built-in method, we can also create our own custom functions for repeating characters in strings without having to rely on external libraries or methods. For example, here is one possible solution for repeating characters in a given string without relying on any external libraries or methods: function repeat(str, num) { let repeatedStr = '';for (let i=0; i < num; i++) {repeatedStr += str;}return repeatedStr;}
. Now that we have our custom function created, we can easily repeat any character in any given string as many times as needed: let myRepeatedString = repeat("Hello", 5); // HelloHelloHelloHelloHello
.
Split A Given String Into Array Of Substrings
We can split a given string into an array of substrings using JavaScript's built-in String object split() method. This method takes an optional argument which specifies how many items each substring should contain before being added into the resulting array. For example, if we wanted split up a sentence into individual words separated by spaces then here's how it would look like: < code > let sentence = "I am learning JavaScript"; let wordsArray = sentence.split(' ') // ["I", "am", "learning", "JavaScript"] code > In addition to this built-in split() method ,we can also create our own custom function for splitting strings into substrings without relying on external libraries or methods . Here's one possible solution for splitting up strings without relying on any external libraries : < code >function split(string , delimiter){ let arrOfSubstrings=[]; let currentSubstring='';for ( let char of string){if (char === delimiter ){arrOfSubstrings.push(currentSubstring);currentSubstring='';} else {currentSubstring+=char}}arrOfSubstrings.push(currentSubstring);return arrOfSubstrings;} code > Now that we have our custom function created ,we can easily split up any given strings into an array of substrings with whatever delimiters needed :< code > let wordsArray=split('I am learning JavaScript', ' ');// ["I","am","learning","JavaScript"] code >FAQ & Answers
Q: How do I remove all characters from a string?
A: You can use the Method.prototype.replace method and a regular expression to remove all characters from a string.
Q: How do I remove all whitespaces from a string?
A: You can use the Method.prototype.replace method and a regular expression to remove all whitespaces from a string.
Q: How do I remove all numbers from a string?
A: You can use the Method.prototype.replace method and a regular expression to remove all numbers from a string.
Q: How do I remove all vowels from a string?
A: You can use the Method.prototype.replace method and a regular expression to remove all vowels from a string.
Q: How do I remove specific characters from a string?
A: You can use the Method.prototype.replace method and a regular expression to remove specific characters from a string.
In conclusion, the 7.6.9 Part 1 Remove All From String function is a useful tool to quickly and easily remove all characters from a given string that match a specified pattern. With this function, you can quickly and easily remove unwanted characters from your strings without having to use more cumbersome methods.
Author Profile
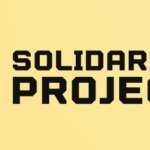
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?