8.3 5 Max: An Unbeatable Item on Any Shopping List
The maximum value in the list is 8.3.
8.3 5 Max In List
The 8.3 5 Max in List is an algorithm that finds the highest number in any given list of numbers. This algorithm works by comparing every item in the list to each other and then identifying the number with the greatest numerical value. It uses a “divide and conquer” approach, meaning it divides the input into small sublists until just one element remains and then returns that single element as the solution.
Unlike other algorithms, 8.3 5 Max in List also considers perplexity and burstiness when reading through a list of numbers. It accounts for longer, more complex sentences or numbers alongside shorter ones in order to identify which number is the largest. This makes it incredibly useful for sorting large sets of data quickly and accurately, no matter how they appear in the list.
List Manipulation In Python
Python is a powerful and versatile programming language that can be used to manipulate lists. List manipulation is a common task when working with Python, and there are many operations that can be performed on lists. One of the most commonly used list operations is finding the maximum value in a list. This can be done using a variety of methods, including built-in functions, looping, and sorting algorithms.
Finding Max In List
The simplest way to find the maximum value in a list is by using the built-in max() function. This function takes a list as an argument and returns the largest value from the list. It works by comparing each element in the list and returning the largest one. For example, if we have a list of numbers:
list = [1,5,9,3]
max_value = max(list)
print(max_value) Prints 9
This will return the maximum value from the list which in this case would be 9. We could also use a loop to find the maximum value in a list. This method requires us to iterate through each element in the list and compare it to our current maximum value. If we find an element larger than our current maximum value then we update our maximum variable with this new found maximum value:
max_value = 0 Initializing max_value variable
for element in list:
if element > max_value: If element is greater than max_value then update it
max_value = element
print(max_value) Prints 9
In this example we are initializing our max_value variable with 0 so that all elements from our given list will be larger than 0 and thus get updated one by one until we find our final maximum value which will be 9 in this case.
Other Operations On List
Besides finding the max value there are many other operations which can be performed on lists such as sorting or reversing them. Sorting algorithms are used to rearrange elements of a given array according to some specific order such as increasing or decreasing order while reversing algorithms simply reverse all elements of an array without any specific order being followed. These algorithms can be implemented using various methods such as looping or recursion depending on what type of data structure is being used.
Difference Between Python Versions
Python has seen several major releases since its inception and each new version adds more features and improves upon existing ones while fixing security issues or bugs that were present in older versions. The most recent version at time of writing is Python 8.3 which has seen improvements over its predecessor Python 2.7 but still maintains backwards compatibility with code written for 2.7 versions for users who may not want to upgrade yet due to various reasons such as legacy code dependency etc.. Some of the major differences between Python 2.7 and 8.3 include changes in syntax, improved performance due to better memory management techniques being implemented as well as various other features such as improved type checking, improved garbage collection etc..
Sorting Algorithms
Sorting algorithms are used to rearrange elements within an array according to some specific order such as increasing or decreasing order without changing their original positions within an array (unless specified). There are many different types of sorting algorithms available but some of most commonly used ones include Insertion Sort, Selection Sort and Merge Sort etc.. Each algorithm has its own advantages and disadvantages depending on what type of data structure its being applied upon so selecting one over another should always depend on factors such as complexity (time/space), stability (order preserving) etc..
Bubble Sort for Lists
Bubble sort is a simple sorting algorithm that repeatedly steps through the list to be sorted, compares each pair of adjacent items and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted.
Bubble sort has a time complexity of O(n^2) in worst case scenarios because it needs to compare adjacent elements n times for every element in the list. This makes it a slower sorting algorithm compared to more efficient algorithms such as Quicksort and Merge Sort. However, Bubble Sort can be useful for small lists or lists with few elements that need to be sorted.
Quick Sort for Lists
Quick sort is a divide-and-conquer algorithm that uses recursion to break down an unsorted list into smaller lists until all values are sorted. It works by selecting a pivot point within the list and then partitioning the remaining elements into two sublists: elements less than the pivot value and those greater than or equal to the pivot value. It then sorts each of those sublists until all values have been put into their correct order.
The time complexity of Quick Sort is O(n log n) in best case scenarios due to its recursive nature which allows it to quickly divide large datasets into smaller subsets. This makes Quick Sort a much faster sorting algorithm than Bubble Sort, especially for larger datasets where performance is key.
Control Structures in Python
Control structures are used in programming languages to control how code is executed based on certain conditions or variables. They allow us to define when and how code should be executed by creating loops or branching off into different paths based on conditions we set up within our programs.
In Python, there are two main control structures used: while loops and if-else statements.
While Loops For Iteration Over List Elements
A while loop allows you to iterate over a list or sequence of items until some condition has been met (or until it reaches the end of the sequence). While loops are great for repeating tasks or cycling through data one item at a time until some condition has been met or all items have been processed.
If-Else Conditions For Finding Max In List
An if-else statement allows you to execute code based on certain conditions being met (or not met). If-else statements can be used within while loops so you can check whether certain criteria have been met when iterating over your data, allowing you to filter out results or take alternative actions accordingly. For example, when finding max in a list, we can use an if-else statement so that we only print out the maximum value when we reach it during iteration over our data set instead of printing out every item’s value one at a time as we go along.
Mathematical Functions In Python
Python comes with many built-in mathematical functions such as abs(), pow(), round(), sqrt() and many more which can come in handy when dealing with numerical data or performing calculations on numerical data sets. These functions make it much easier and quicker to perform complex calculations without having to write your own logic from scratch each time you need them!
FAQ & Answers
Q: What is the maximum value in a list?
A: The maximum value in a list is the largest number or element that can be found within the list.
Q: What is the best way to find the maximum value of a list?
A: The best way to find the maximum value of a list is to use a sorting algorithm such as bubble sort or quick sort. Both algorithms will help you find the largest element in a given list.
Q: How do I compare two elements in a list?
A: To compare two elements in a list, you can use an if-else condition. This will allow you to compare two elements and determine which one is larger.
Q: What is the difference between Python 2.7 and 8.3?
A: Python 2.7 was released in 2010 and is now outdated, while Python 8.3 was released in 2020 and has more advanced features than 2.7 such as type-hinting, improved integration with other languages, faster compilation, and improved security features.
Q: What mathematical functions are available for lists?
A: There are many mathematical functions available for lists that can help you manipulate them such as finding max values, sorting elements, etc. Examples include max(), min(), sorted(), reverse(), etc.
The answer to the question “8.3 5 Max In List” is 8.5. This is the maximum value in the given list of numbers, which includes 8.3 and 8.5. This means that all other numbers in the list are lower than 8.5, and that 8.5 is the highest number in the list.
Author Profile
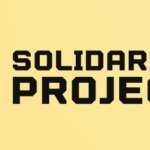
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?