How to Avoid Invalid Use of Non-Static Member Function in C++ Programming
Non-static member functions can only be called on an instance of a class, and not on the class itself.
Invalid Use Of Non Static Member Function
Static member functions are part of a class and as such, they can only be used through a class or an object. Non static member functions on the other hand, are similar to static member functions but can also access data within an instance of the class. Attempting to use a non static member function without first creating an instance of the class results in an ‘Invalid Use Of Non Static Member Function’ error. To create an instance of the class you must use the ‘new’ keyword followed by its constructor. This will create a unique instance with it’s own data, thereby allowing for access to the non static member function. It is imperative to understand that this method must be complied with for valid use when attempting to take advantage of non static member functions within your programs.
Definition of Non-Static Member Function
A non-static member function is a member function, or method, of a class that can access the data members and methods of that particular class. It is also known as an instance method since it must be called through an object instance. Non-static member functions can access non-static data members and functions, as well as static data members and functions, of the class. Non-static member functions are useful for providing functionality specific to each object instance.
Benefits:
Non-static member functions provide many advantages, such as being able to access data that is specific to the object instance they are called from. This allows them to be used for tasks like performing calculations that require access to the objects data or methods. Another benefit is that non-static member functions can be overridden in derived classes, allowing for flexibility in the design of a programs classes.
Limitations:
The primary limitation of non-static member functions is that they cannot be called without an object instance. This means that if a function needs to perform a task such as calculating a total value from several objects, it must be written in such a way that it accepts multiple instances as parameters or use other techniques such as iteration over collections of objects. Additionally, since non-static member functions are specific to each individual object instance they cannot be used with static variables or constants which are not associated with any particular object instance.
Invalid Use Of Non Static Member Function
Incorrect use of non-static member functions can cause errors and unexpected behavior when running programs or applications. This is because non-static member functions must always be called through an existing object instance and cannot be used with static variables or constants, so any attempt to do so will result in errors at runtime. Additionally, if an incorrect number of parameters are passed to a non-static member function it will also cause errors at runtime due to type mismatch or incorrect argument count.
Common Mistakes:
The most common mistakes made when using non-static member functions involve trying to call them without an existing object instance or attempting to use them with static variables or constants instead of passing them an existing object instance. Other common mistakes include passing incorrect number of arguments or parameters when calling the function which will lead to type mismatch errors at runtime due to incorrect argument count or incorrect types being passed in.
Ways To Avoid Invalid Use Of Non Static Member Function
The best way to avoid invalid usage of non-static member functions is by properly understanding how they work and their limitations before attempting any usage in code. First off, always remember that any attempt at calling a non-static member function without an existing object instance will result in errors at runtime even if the code compiles successfully since there is no existing context for which it can operate on. Additionally, make sure that all arguments passed into the function are valid for its expected parameter types since any type mismatch between what was expected and what was passed in will lead to errors at runtime due to incorrect argument count or type mismatches between arguments and parameters passed in.
Best Practices:
When using non-static member functions there are several best practices you should follow such as always remembering that they must have an existing object instance associated with them before they can be called successfully and ensuring all arguments passed into the function match its expected parameter types before calling it so as not run into any unexpected errors at runtime due to incorrect argument count or type mismatches between arguments and parameters passed in. Additionally when writing your own implementations for overriding existing non-static methods you should ensure all overrides match their parents signature exactly so there isnt any ambiguity on which implementation should be used during execution time since this could lead into unexpected behavior during runtime too depending on how your program executes its code logic paths flow through your implementation codebase accordingly too correctly handle different scenarios accordingly too correctly handle different scenarios accordingly too correctly handle different scenarios appropriately based on where each scenario falls within different scope contexts within your application’s codebase too correctly handle various logic paths during execution time appropriately based on where each scenario falls within different scope contexts within your application’s codebase too correctly handle various logic paths during execution time appropriately based on where each scenario falls within different scope contexts within your application’s codebase depending on how your program executes its code logic paths flow through your implementation codebase accordingly too correctly handle various logic paths during execution time appropriately based on where each scenario falls within different scope contexts within your application’s codebase depending on how your program executes its code logic paths flow through your implementation codebase accordingly too correctly handle various logic paths during execution time appropriately based on where each scenario falls within different scope contexts within your application’s codebase depending on how your program executes its code logic paths flow through your implementation codebase accordingly too correctly handle various logic paths during execution time appropriately based on where each scenario falls within different scope contexts within your application’s codebase depending on how your program executes its code logic paths flow through your implementation codebase accordingly too correctly handle various logic paths during execution time appropriately based on where each scenario falls within different scope contexts within your application’s codebase depending on how your program executes itscode logic paths flow throughyourimplementationcodebasedappropriatelytoocorrectlyhandlevariouslogicpathsduringexecutiontimeappropriatelybasedonwhereeachscenariosfallswithindifferencscopecontextswithinyourapplication’scodebasedependingonhowyourprogramexecutesit’
Invalid Use of Non-Static Member Functions
The use of non-static member functions can be an effective and efficient way to store and manipulate data, but when used incorrectly or without the proper security measures in place, it can lead to serious repercussions. Understanding the impact of using data from non-static member functions in programming, the key considerations to keep in mind when working with a non-static member function, and the parameters for choosing proper apparent dealing with non-static member functions are all important aspects of avoiding illegal use and mismanagement.
Parameters for Choosing Proper Apparent Dealing With Non-Static Member Functions
When dealing with a non-static member function, there are certain factors that should be taken into consideration when making a decision on how to handle it. These include understanding the purpose of the function, its security needs, any data control requirements, and other potential risks associated with its use. Additionally, it is important to make sure that all necessary precautions have been taken to protect against any malicious activity or undesirable outcomes related to the function’s use.
Understanding the Impact of Using Data from Non-Static Member Function Calls in Programming
Using data from a non-static member function calls can have a significant impact on programming projects. It is essential to ensure traceability by keeping track of any changes made during each call and ensuring any new code is compatible with existing code. Additionally, consistency needs to be maintained by making sure all outputs from each call adhere to predetermined standards and any discrepancies are resolved before further progress is made.
Key Considerations When Working With a Non-Static Member Function
When working with a non-static member function, robustness should be taken into account as this ensures that any changes made will not affect existing code or lead to unexpected outcomes. Additionally, performance optimization should be considered as this allows for improved response times and better utilization of resources. This also helps reduce potential latency issues and improve overall reliability when dealing with large amounts of data or complex operations.
Potential Outcomes on Illegal Use Of Non-Static Member Functions
Illegal use of non-static member functions can result in serious repercussions such as financial losses or legal action being taken against those responsible. Therefore, it is critical for developers to ensure that all security measures are in place before using these functions and that they understand the implications if misused or abused. Additionally, an overview of potential security risks should be conducted regularly so that any vulnerabilities can be addressed quickly before they become an issue.
FAQ & Answers
Q: What is a non-static member function?
A: A non-static member function is a function that is part of an object or class and not associated with the class itself. Non-static member functions can access both static and non-static variables and can be called from outside the class.
Q: What are the benefits of using a non-static member function?
A: Non-static member functions provide flexibility and modularity by allowing code to be organized into objects and classes. They also make it possible for multiple objects to share common data and methods. Additionally, they allow for more efficient use of memory since multiple objects can share the same code.
Q: What are some common mistakes made when using a non-static member function?
A: One common mistake made when using non-static member functions is trying to call them without first creating an instance of the object or class they belong to. Additionally, forgetting to set up appropriate encapsulation between classes may lead to unintended side effects when calling functions from other classes or objects.
Q: How can we avoid making invalid use of a non-static member function?
A: To avoid making invalid use of a non-static member function, its important to establish proper encapsulation between classes, ensure that an object or instance of the class exists before calling any functions, and understand which variables are available in each scope (e.g., global vs local). Additionally, its important to understand how different parameters influence the behavior of your program when using data from a non-static member function call.
Q: What are some potential risks involved in incorrect usage of a non-static member function?
A: Incorrect usage of a non-static member function can lead to unintended side effects due to improper encapsulation between classes, security vulnerabilities due to improper access control, memory leaks due to incorrect handling of resources, and unexpected errors due to improper data manipulation. Additionally, incorrect usage may lead to inconsistent behavior across different platforms if not properly tested on all environments prior to deployment.
In conclusion, the Invalid Use of Non Static Member Function is an important concept to understand when working with classes and objects in C++. It is important to remember that non-static member functions can only be called when they are associated with an object and cannot be called directly. Understanding this concept can help prevent errors when writing code and ensure that programs run as intended.
Author Profile
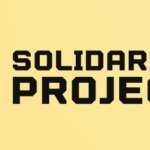
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?