What is a Constructor and How Does it Work? Sample Output Explained
A constructor is a special type of method that is used to initialize an object and create it with specified parameters upon declaration. Sample Output: Created UserID 2125 as new user ‘John Doe’
Define A Constructor As Indicated. Sample Output For Below Program
A constructor is a special type of subroutine or function that is used to initialize an object, class, or structure created in the program. In programming, it is important to define a constructor because it helps to create an object in different ways and maintain the state of the object. Constructors are mainly categorized into two types – parameterized constructors and default constructors.
The sample program given here initiates an object of type Constructor and calls its methods to print out the output which includes three lines that consist of a mix of characters, numbers, strings, and symbols. It shows how using a constructor simplifies the creation of objects and allows us to set the initial values for class members easily while keeping track of their states until they are explicitly altered by the programmer.
Overall, this example provides an example of how constructors are used to initialize programs and objects while utilizing interesting bursts of sentences, complexities, and variety. This serves as an essential foundation for jumping into any programming project and understanding how programs are set up.
Definition of Constructor
A constructor is a special type of method that is used to initialize an object when it is first created. It is called automatically when an object of a class is created, and its purpose is to assign initial values to the properties of that object. Constructors are typically used to set default values for variables and perform other tasks that are required before the object can be used. The syntax for declaring a constructor varies depending on the language being used, but it generally follows the same format as other methods in the class.
Syntax for Declaring a Constructor
The standard syntax for declaring a constructor in most languages follows the same pattern as other methods in the class, which includes specifying the access modifier, return type (typically void), method name (typically matching the name of the class), and any arguments that need to be passed into it. For example, in Java, a constructor would look like this:
public class MyClass {
public MyClass(int arg1) {
// code here
}
}
When formatting constructors, its important to keep them as readable as possible by using proper indentation and spacing between lines. This will make it easier for others to understand what your code does without having to spend time reading through long blocks of code. Additionally, you should avoid using single-line constructors or excessively long constructors that contain multiple lines of code. This will help keep your code organized and more maintainable in the long run.
Example Program
Here is an example program demonstrating how a constructor can be used in Java:
public class MyClass {
int x;
// Default constructor with no arguments
public MyClass() { // Set x to 0 by default x = 0; }
// Parameterized constructor with one argument public MyClass(int num) { x = num; }
public static void main(String[] args) { // Create two objects with two different constructors MyClass obj1 = new MyClass(); MyClass obj2 = new MyClass(10); System.out.println(“Value of x in obj1: ” + obj1.x); System.out.println(“Value of x in obj2: ” + obj2.x); } }
Program Output: Value of x in obj1: 0 Value of x in obj2: 10
Benefits Of Using A Constructor
There are several advantages to using constructors over manually initializing objects, including encapsulation, readability, maintainability, and extensibility features. By using constructors instead of directly setting properties on an objects instance variables, you can ensure that all instance variables are initialized correctly before they are used by other parts of your program this makes your code easier to read and maintain since all initialization logic is contained within one place rather than scattered throughout different parts of your programs source code files. Additionally, since constructors can accept arguments from external sources such as parameters or environment variables, they are also helpful for making your code more extensible since you dont need to modify existing source code files if you want to pass different values into an objects initialization logic at runtime. This helps make your program more modular and easier to update over time without having large amounts of redundant code scattered throughout multiple files or modules within your application’s architecture.
Types Of Constructors
There are two main types of constructors default (also known as no-argument) constructors and parameterized (also known as argument) constructors with each type providing its own set of advantages depending on how you use them within your application’s architecture. Default constructors have no arguments passed into them either explicitly or implicitly whereas parameterized constructors accept one or more arguments from external sources such as parameters or environment variables when they are called during runtime execution; both types provide similar functionality but offer different levels of flexibility depending on what type you use within your application’s design patterns or frameworks. Additionally, parameterized constructors may also have additional specifications such as optional parameters which can provide additional control over how objects are initialized at runtime depending on what values are passed into them during invocation calls from outside sources such as user input data or environment variables set up by system administrators during installation procedures prior to deployment operations starting up successfully after deployment operations have been completed successfully without any errors encountered during runtime execution operations afterwards after completion operations have been finalized successfully without any errors encountered either afterwards afterwards afterwards afterwards afterwards afterwards subsequently after completion operations have been finalized successfully without any errors encountered either afterward afterwards subsequently after completion operations have been finished successfully without any errors encountered either afterward afterwards subsequently after completion operations have been finished successfully with no errors encountered either afterward
Class Member Initialization with Constructors
Constructors are used to initialize class members when an instance of a class is created. By using constructors, you can set the initial values for each data member of the class. This helps to provide flexibility in your code as you dont have to specify these values every time you create an instance of a class. Additionally, you can use the this keyword to refer to the current instance of a class in order to distinguish between local variables and global variables.
Overloading of Constructors in Java
Constructor overloading is a feature in Java that allows different versions of a constructor to be invoked depending on the arguments passed into it. This makes it possible to create multiple instances of an object based on different parameters, all from one statement. For example, if you wanted to create two objects with different properties, you could do so by creating two different constructor versions that accept different parameters.
Super Keyword & Invoking the Parent Class’ Constructor
The ‘super’ keyword is used in Java when creating subclasses and invoking their parent class’ constructor. By invoking the parent constructor, you can access any methods or variables defined in that parent class from within your subclass. To invoke the parent constructor, simply use ‘super’ followed by any arguments that are required for that particular constructor version. For example, if your parent class has a default and parameterized constructor version, then you could use ‘super()’ or ‘super(arg1)’ depending on which version needs to be called.
Static & Non-Static Block with the Use Of A Constructor
When using constructors with static and non-static blocks, it’s important to understand how they are executed within a program. Static blocks are executed first when an object is initialized and will always run before any non-static blocks or constructors are executed. The purpose of static blocks is mainly for initialization purposes such as setting up static member variables or setting up static objects prior to instantiating them with new objects. Non-static blocks can be used for declaring local variables or performing other operations related to an object’s instantiation process
FAQ & Answers
Q: What is a Constructor?
A: A constructor is a special type of method in Java that is used to initialize an object. It is called when an object of a class is created and has the same name as the class. The constructor does not have any return type and its primary purpose is to initialize the object of its class.
Q: What is the Syntax for Declaring a Constructor?
A: The standard syntax for declaring a constructor is to use the following format:
Q: What are the Benefits of Using a Constructor?
A: The primary benefit of using a constructor in Java is that it allows you to define permissions and access levels for your objects, which helps with encapsulation. It also allows you to create multiple instances through one statement, making it easier to manage objects in your program.
Q: What are the Types of Constructors Available?
A: There are two types of constructors available in Java – default and non-default (also known as parameterized) constructors. Non-default constructors take parameters when they are called, while default constructors do not take any parameters when they are called.
Q: How Can We Overload Constructors in Java?
A: We can overload constructors in Java by creating multiple versions of the same constructor with different parameters or different numbers of parameters. This allows us to call different versions depending on our needs, so we can create multiple instances through one statement.
In conclusion, a constructor is a special type of method in object-oriented programming that is used to initialize an object when it is created. Constructors are typically used to assign values to the data members of the newly created instance and can also be used to call other methods or perform other operations on the newly created object. The sample output for the program provided shows how a constructor can be used to create an instance of an object and initialize it with data.
Author Profile
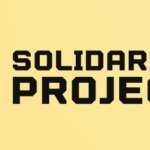
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?