What Is Out Of Line Definition in C++? An Overview With Keywords
Out of line definition C++ is when a function definition appears outside the scope of Class.
Out Of Line Definition C++
Out-Of-Line Definition C++ is a feature of the C++ programming language that allows users to define certain objects or functions within the scope of the program. This means that these objects or functions can be declared and defined in any place of the program even if they are located outside the main class, structure, union, parameter list, or a return type. This feature helps in improving memory usage and performance as it ensures that an object is allocated only once and all references point to the same object. Out-Of-Line Definition can also help simplify complex notebooks by allowing multiple files with different sections that are less cluttered. Furthermore, it maintains classes’ encapsulated state by virtue of restricting access as no one can define something within another class. Overall, Out-Of-Line Definition C++ offers users a unique way to manipulate and work with data within their programs and helps provide them with better control over their code.
Out Of Line Definition C++
Definition
Out-of-line definition (OOL) is a concept in the C++ programming language that allows a programmer to define function members outside of the class definition. This is useful when the class definition is long and complex, or when declaring multiple functions within a single class. By defining functions outside of the class definition, it allows for greater readability and organization of code. Additionally, OOL can help reduce compile time by pre-processing code prior to compilation.
Examples
An example of OOL would be declaring a function within a class as follows:
Class MyClass {
public:
void doSomething(); // Declaring the function here
};
Then defining that same function outside of the class as such:
void MyClass::doSomething() { // defining the function here }
This allows the programmer to break up complex functions into multiple parts, making it easier to debug and maintain. It also reduces compile time by pre-processing code prior to compilation.
Benefits
The main benefit of using OOL is that it allows for greater flexibility and readability in complex programs. By separating out large functions from the main class definition, it makes it easier for other programmers to identify what each part of the program does without having to go through an entire block of code for every single line. Additionally, it helps with debugging as individual lines can be identified more easily if they are separated from one another. Finally, OOL helps with compile time by pre-processing code before compiling it into an executable program.
Limitations
Although OOL offers many benefits, there are some limitations that should be considered before using this approach in programming. For example, if multiple functions are declared within one class then all of those functions must be defined outside of the class in order for them to work properly; this can lead to increased complexity and confusion when trying to debug or maintain a program. Additionally, if too many functions are declared outside of a single class then memory usage may become an issue since all declared functions will need their own allocated memory space for proper functioning.
Syntax
In order to use OOL properly in C++ programming there are two main syntax rules governing how functions should be declared and defined:
Declaring Function Members Outside The Class
When declaring function members outside of a class they should follow this syntax rule: return_type Class_name::function_name(parameters) { // body }
Implementation Of Defined Functions
When implementing defined functions they should follow this syntax rule: return_type Class_name::function_name(parameters) { // body return value; }
Memory Allocation
When using out-of-line definitions in C++ programming it is important to consider memory allocation requirements for each defined function member since each will need its own allocated space in memory for proper functioning. There are two primary techniques used for allocating memory space: static allocation and dynamic allocation.Memory Requirements h3 > Static allocation requires that all required memory space be allocated prior to execution whereas dynamic allocation requires that only enough memory space needed at any given time be allocated at any given time which can help reduce overall memory usage requirements depending on certain application requirements.
< h3 > Memory Allocation Techniques h3 > When using static allocation techniques all required memory must be allocated upfront whereas dynamic allocation techniques require only enough memory needed at any given time which helps reduce overall memory usage requirements depending on certain application requirements but can cause performance issues if not implemented properly due to unnecessary reallocations or deallocations occurring during runtime when not necessary which can lead to decreased performance or even crashes if done incorrectly or too frequently over an extended period of time due to running out of available system resources such as RAM or CPU cycles etcetera so care must be taken when implementing these types of optimization techniques within programs utilizing out-of-line definitions in order ensure optimal performance without sacrificing stability or security considerations .
< h2 >Different Syntax Rules Governing Out Of Line Definitions h 2 > There are two primary syntax rules governing out-of-line definitions which include Function Declaration vs Definition as well as Scope Of Data Member Variables .
< h 3 >Function Declaration Vs Definition h 3 > When declaring functions outside of classes they should follow this syntax rule : return _ type Class _ name :: function _ name ( parameters ) { // body } . This ensures that all necessary data member variables have been declared before attempting implementation so that no errors occur during runtime dueto variables being accessed etcetera .
< h 3 >Scope Of Data Member Variables h 3 > When defining data members within classes their scope should also be considered carefully since data member variables defined within the scope will only have access rights till they remain within their respective scopes . Any access attempts from different scopes will result in errors unless properly handled beforehand via appropriate mechanisms such as public / private / protected modifiers etcetera .
Dependency between Declaration and Definitions in C++
In the C++ programming language, the relationship between a declaration and its corresponding definition is referred to as visibility rules. These rules dictate how variables, functions and other objects are accessed by other parts of the code. The binding theory between a declaration and its definition is an important concept in C++ programming. It describes how a given object is visible to another part of the code, as well as how it is stored in memory.
The visibility rules are defined by two main principles: the scope of a given object, and the linkage of that object. The scope of an object defines where it can be accessed from within a program, while the linkage determines whether or not multiple definitions of an object can exist at one time. By understanding these principles, programmers can design better programs that are easier to maintain and debug.
Code Performance and Optimization with Out Of Line Definitions in C++
Out-of-line definitions enable optimization techniques that can dramatically improve code performance metrics such as execution time, memory usage and object file size. When out-of-line definitions are used, functions can be split into multiple pieces which allows for better optimization opportunities since each piece can be optimized independently from each other. This also allows for more reuse of common code segments since they do not need to be re-written every single time they are used. Furthermore, this reduces the size of an object file since only one copy of each function needs to be included instead of multiple copies if inline definitions were used instead.
Best Practices for Writing Better Code with Out Of Line Definition
When using out-of-line definitions, there are several best practices that should be followed in order to write more efficient and maintainable code. First, all function definitions should be placed outside of any class or struct declarations when possible. This helps reduce clutter inside class/struct declarations which makes them easier to read and understand. Second, good coding style should always be followed when writing out-of-line function definitions; this includes using clear variable names as well as consistent formatting throughout your codebase. Finally, memory efficiency should always be taken into account when writing out-of-line function definitions; this includes avoiding unnecessary data structures or data copies whenever possible and using appropriate data types for each variable/function parameter depending on the situation at hand.
FAQ & Answers
Q: What is Out-of-Line Definition in C++?
A: Out-of-line definition is a technique in C++ which allows the definition of class members outside of the class declaration. This technique helps to separate the interface and implementation details making the code more organized and readable. It also allows developers to make changes easily without having to modify the class declaration.
Q: When to use Out-of-Line Definition?
A: Out-of-line definition should be used when a member function requires a large amount of code or when there are multiple overloaded versions of the same function. It is beneficial to use this technique as it allows for better organization and readability of code, and makes it easier to maintain and debug.
Q: What is the Syntax for Out-of-Line Definition in C++?
A: The syntax for out-of-line definition involves declaring a function member outside of the class declaration, followed by an implementation of the defined functions. This can be done by using either an inline or non-inline syntax depending on whether you want all definitions within one file or split across multiple files.
Q: What is Memory Allocation for Out-of-Line Defined Function?
A: Memory allocation for out-of line defined functions depends on how much memory each function requires. The memory requirements vary depending on how complex or lengthy your code is. Memory allocation techniques such as dynamic memory allocation can be used to allocate sufficient memory for out of line defined functions.
Q: What are some Different Syntax Rules Governing Out Of Line Definitions?
A: Different syntax rules governing out of line definitions include differentiating between function declarations and definitions, as well as understanding the scope of data member variables available in different scopes within a class declaration. Additionally, understanding dependency between declarations and definitions in C++, such as visibility rules and binding theory between declaration and definitions, will help developers write better code with out of line definition techniques.
In conclusion, the out of line definition in C++ is a way to define a function outside of a class declaration, without having to write it in the header file. This provides more flexibility when making changes to the code, as well as allowing for better memory management and better performance. Out of line definitions are an important concept to understand when working with C++.
Author Profile
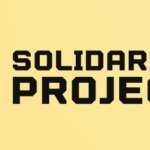
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?