Learn to Create Lab Mad Libs with Loops: A Step-by-Step Guide
The for loop is perfect for executing a set of instructions a certain number of times.
4.17 Lab Mad Lib – Loops
The 4.17 Lab Mad Lib Loops is an educational tool that provides a great way for students to learn programming fundamentals. It uses the creative writing game known as ‘Mad Libs’ to teach users the basics of looping structures, which are fundamental mathematical operations that allow loops to repeat certain parts of a program. Through a series of straightforward instructions and exercises, users are able to acquire basic coding skills such as interpreting loop conditions and manipulating sentinel values. The language used within the game is straightforward but intricate enough to enable learners to get accustomed to loop programming fundamentals that can be applied in future coding endeavors. The complexity level of this program is perfect for introducing novice coders of any age group to the concepts of looped programming without overwhelming them with too much complex syntax at once. With an enjoyable learning experience, the 4.17 Lab Mad Lib Loops offers a great way for students with all levels of coding proficiency to get introduced into looped programming skills and practices.
Introduction to Loops
Loops are code structures that enable a certain section of code to be executed multiple times, in a row. This can be extremely useful in programming tasks, as it allows for more efficient writing and running of code. There are two main types of loop: the for loop and the while loop.
The for loop is particularly useful when iterating through a list, allowing you to access each element in the list one by one. The while loop is more flexible and can be used when you don’t know how many times the code needs to be executed until a certain condition is met.
Executing a While Loop
The syntax of while loops is simple; they consist of a condition check followed by a body of code that will execute as long as the condition is true. The structure looks like this:
“`while (condition){
// Loop body
}“`
In order to execute a ‘for each’ iteration with a list, you would set up your while loop like so:
“`int index = 0;
while (index < list.size()) { //Loop body index++; }``` Here, we have declared an integer named 'index' which acts as an iterator for the list and allows us to access each element in turn. We then set up our while loop with the condition that 'index' must be less than the size of the list - this ensures that we won't try to access an element that doesn't exist in our list! The body of our loop will contain whatever code we wish to execute on each element in our list, and finally we increment 'index' so that it points to the next element in our list each time our loop executes.
Using Break Statement in Loop
Break statements are used within loops when we want to terminate execution prematurely – i.e., if some condition has been met before reaching the end of our loop body that means it’s no longer necessary for us to continue executing any further iterations. This can help make code more efficient by avoiding unnecessary iterations, saving both time and memory!
Identifying when break statements are applicable requires careful consideration of what conditions need to be satisfied for your particular task – if there are multiple possibilities or conditions then you’ll need multiple break statements within your loop body! For example, if you were searching through a sorted array for some specific value then you could use a break statement once you had found it so that any further iterations would become redundant.
Working with Strings
When working with strings, loops can prove incredibly useful as they allow us to easily print out each character in succession or concatenate several strings together into one resulting string without having to manually type out every single character or string separately! In order to print out characters from a string using a loop we would first declare an integer variable named ‘i’, which will act as our iterator over the string:
“`int i = 0;
while (i < str.length()) {
System.out.println(str[i]); //print out character at index i
i++; //increment iterator so next iteration moves on one character
}```
To concatenate two strings together using loops we would set up something similar:
```String newStr = ; //declare empty string variable which will hold result
int i = 0; //declare iterator variable
while (i < str1.length()) { //loop over first string
newStr += str1[i]; //add current character onto end of newStr
i++; //increment iterator so next iteration moves on one character
}
i = 0; //reset iterator back to 0
while (i < str2.length()) {//loop over second string
newStr += str2[i];//add current character onto end of newStr
i++;//increment iterator so next iteration moves on one character
}``` ` ` ` ` ```````````````````````````````` `` ```` `` ` ` ` ` ` `` `` `` `` `` ` ` ` ` ` ` `` `` `` `` `` `` ` ` **Manipulating Lists by Looping Through Them** Manipulating lists can also be done using loops which makes life much easier than trying to do it manually! For example suppose you wanted select certain values from your list and modify them - this could easily be done using loops:
```for(int i=0; i
int temp = list[i+1];
list[i+1] = list [i];
list[i] = temp;
}
}
Counting Instances of Things
Counting instances of things in a list or string can be a difficult task. Doing so involves searching for elements and their frequency present in the list or string, as well as finding specific characters or words from the string or list. This can be done using a variety of methods, such as using for loops and while loops to iterate through the list and count the number of times an element appears. Additionally, there are several built-in functions that can be used to count elements in a list, such as the len() function for strings, or the count() function for lists.
Nesting Loops
Nesting loops is a method of looping that is used to iterate through multiple data structures simultaneously. This technique allows for the mutation of values from nested loops, allowing for more complex operations than would normally be possible with non-nested loops. When nesting loops it is important to ensure that they are properly indented according to best practices, such as using 4-space indentation standards, 2 spaces for indentation, and properly indenting each line 4 spaces. Additionally, it is important to maintain indentation consistency across codes when nesting loops in order to avoid errors and unexpected results.
Differentiating Between Nested and Non-Nested Loops
In order to differentiate between nested and non-nested loops it is important to understand their inter connectedness. Nested loops are those which contain one loop inside another loop – this allows for more complex operations than would normally be possible with non-nested loops. Non-nested loops on the other hand do not contain any additional loop within them – they simply run through each item in a given data structure until all items have been processed. Knowing when and where to use either type of loop is essential for efficient programming.
Encoding/Decoding Endings
When encoding/decoding endings it is important to use special characters in order to limit while loops and extract values on each loop iteration. For example, adding \ at the end of each line can help limit while loops so that they process only up until that character has been reached rather than processing an entire large file all at once. Additionally, adding brackets around certain values on each iteration can help extract those values more easily from within a while loop since they will stand out from other elements within the loop.
FAQ & Answers
Q: What are loops?
A: Loops are a set of instructions that can be repeated multiple times in order to automate tasks. Loops can be used to iterate through a list of items, or they can be used to execute a set of instructions until a certain condition is met.
Q: What are the different types of loops?
A: The two most common types of loops are for and while loops. For loops iterate through a list of items, while while loops continuously execute instructions until the specified condition is met.
Q: When should I use break statements in my loop?
A: Break statements should be used when you want to exit out of the loop early, usually when a certain condition has been met. This can be useful if you want to stop executing the loop at a certain point or if you need to skip over certain values.
Q: How do I loop through strings and lists?
A: To loop through strings and lists, you can use for each iterations with a list or you can use while loops with specific conditions. You can also use nested loops in order to manipulate values from nested lists.
Q: What are some best practices for code indentation when using loops?
A: It is important to maintain consistent indentation across your code when using loops so that it is easy to read and follow. The best practice for indentation when writing code is to use 4-space indentation standards and add multiple indents for each heading and subheading within the code.
In conclusion, Loops are an essential tool for programming and can be used to greatly improve the efficiency of a program. The 4.17 Lab Mad Lib – Loops is a great way to practice using loops in Python and get a better understanding of how they work. With some practice, anyone can become proficient at using loops to create powerful programs.
Author Profile
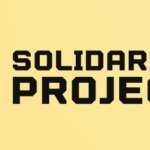
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?