Ensuring Function Overloading Is Distinguished By More Than Just Return Type Alone
No, functions cannot be overloaded solely based on differences in return type.
Cannot Overload Functions Distinguished By Return Type Alone
Cannot Overload Functions Distinguished By Return Type Alone is a concept in programming which states that two functions with the same name, but differing only in return type, cannot be overloaded. This is due to the fact that return types are determined at compile time and are not considered when overloading functions. Thus if you have two functions of the same name with different return types they will be treated as separate entities by the compiler. For example, if you have a function named “doSomething()” which returns an int and a different doSomething() which returns a double, these would be treated as separate functions and not overloaded. To overload such functions means they must have different argument lists or differ only in their return values.
Overloading A Function
Overloading a function is a process of providing multiple definitions for the same function, varying in the types and/or number of parameters it accepts. This allows the same function to be used for different types of data and makes code more concise and easier to read and maintain. The advantage of overloading a function is that it reduces the amount of code writing that needs to be done, as one single function can perform many different tasks.
Identification of Overloaded Functions
Identifying overloaded functions can be challenging because all functions have the same name, but different parameters and return types. To differentiate between the functions, there are several techniques that can be used such as examining parameter types, looking at return type, and checking for any additional data that may be passed in as an argument. Additionally, some programming languages have specific techniques for identifying overloaded functions, such as C++s argument matching feature.
Reasons Behind Not Allowing Execution Of Overloaded Return Types
The primary disadvantage to this method is that it can introduce ambiguity into the code since two functions could potentially have conflicting return types. For example, if two different overloaded versions of a function exist with one returning an integer and one returning a string then it can become difficult to determine which one should be called when needed. As such, this is generally not allowed by most programming languages to prevent confusion or errors from occurring in code execution.
Argument Matching In C++
C++ offers an argument matching technique which allows programmers to easily identify overloaded functions without having to manually check each type of parameter or return type. This method creates a unique signature for each overloaded version of a function based on its parameters and return values. It then uses this signature when attempting to match arguments with their respective definitions. For example, if there are two versions of a function with different parameters but the same return type then C++ will use its argument matching feature to determine which version should be executed based on how closely the arguments match up with each parameter signature.
Specification By Standard Libraries
Standard libraries offer predefined rules for managing return values when dealing with overloaded functions. This ensures consistency across all programs using the library by enforcing certain standards when dealing with ambiguous cases where two or more versions of a function may exist with varying return types or parameters. Additionally, some standard libraries also provide default behaviors for normalizing variables which helps reduce any potential conflicts between multiple definitions in code execution. This helps ensure that programs utilizing these libraries are able to execute properly without any issues related to overloading inconsistency or conflict resolution issues arising during compilation or run time processes.
Implicit Conversion To Achieve Desired Outputs
Implicit conversion is a process of altering the datatype of a variable without explicitly changing it. It is done to achieve the desired output from exploiting weak typing. Weak typing allows an expression to be evaluated with different types, and implicit conversion can be used to make sure the expression has compatible types. For example, if an integer value is taken as input and the desired output is a string, implicit conversion can be used to convert the integer into a string so that the desired output can be achieved.
Implicit conversion can also come in handy when dealing with different data types. For instance, if one variable is an integer and another variable is a float, implicit conversion can be used to convert one of them into the other type so that they are compatible and no errors are generated. This kind of conversion is useful when dealing with complex operations involving multiple data types.
Implication of Constants In Interface Declaration
Constant qualifiers are used in interface declaration when declaring member variables or functions in order to specify that their values cannot change during runtime. This helps ensure that the behavior of the program remains consistent as it should. Additionally, it also helps enforce certain regulations regarding passing arguments and other operations which depend on these constants being defined beforehand.
For instance, let’s say we want to pass an argument as a constant value while declaring a function; we would need to specify this information beforehand using constant qualifiers so that our program remains consistent throughout its execution. Without this information being specified beforehand, our program might produce unexpected results or even crash due to invalid arguments being passed.
Increasing Complexity During Debugging Processes
Debugging processes are often complex due to various underlying factors which contribute towards distortion in the codebase. This includes incorrect usage of data structures or algorithms, incorrect assumptions made while coding, or even incorrect declarations made when defining objects or functions. All these factors add up and make debugging harder than it should be since they all need to be identified and corrected accordingly before progress can be made towards achieving our desired output or fixing any bugs present in our codebase.
Furthermore, debugging processes also consume a lot of time and resources due to constant tuning and tweaking which needs to take place in order for us to get our desired outcome from our codebase. This makes debugging processes even more complex since it not only requires us to identify any underlying issues contributing towards distortion but also requires us to make sure that whatever changes we make do not break anything else within our program before finally reaching our goal of getting rid of any bugs present within our codebase.
Take Away From All Thoughts
When it comes down all thoughts related with overloading functions distinguished by return type alone, there are several key principles which should not be breached under any circumstances such as making sure variables have compatible datatypes before applying implicit conversions etc., Additionally, there’s also an inherent dilemma involved in making error free conversions between different data types as well as ensuring constants are correctly specified before attempting any operation on them during debugging processes – all these factors contribute towards increasing complexity during debugging process which ultimately leads us back into making sure we understand all principles related with overloading functions distinguished by return type alone before attempting any operations on them within our programs in order for us achieve expected outcomes without encountering any errors along the way
FAQ & Answers
Q: What is overloading?
A: Overloading is a feature of the programming language that allows us to create multiple functions with the same name, but with different parameters or arguments. This allows us to use the same function name for different types of data and parameters.
Q: What are the advantages of overloading functions?
A: One advantage of overloading functions is that it makes the code easier to read and understand, as it allows us to use the same name for a function that performs different tasks. It also makes our code more efficient, as it allows us to reuse code and avoid writing multiple functions for similar tasks. In addition, it can also reduce errors in our code, since we can ensure that all functions with similar names have similar behaviors.
Q: How do we differentiate overloaded functions?
A: We can differentiate overloaded functions by their signatures, which include number of arguments, types of arguments and order of arguments. We can also differentiate them by their return type and const qualifier they contain.
Q: What are the implications of constants in interface declaration?
A: Constant qualifiers on member variables can be used in interface declarations in order to limit what values a function or variable can take or return. This helps us ensure that our functions will always be called with valid values and return predictable results. Additionally, it helps reduce errors when calling a function with invalid arguments, as well as making our code more robust and reliable.
Q: What are some potential issues when debugging overloaded functions?
A: One potential issue when debugging overloaded functions is that they may produce unexpected results due to implicit conversion between different types or argument mismatch between different versions of the same function. Additionally, errors may arise from ambiguity in possible solutions due to weak typing or mismatches between argument types and return types. Finally, debugging overloaded functions may cause an increase in complexity due to constant tuning and time spent trying to figure out why certain versions of a function are being called instead of others.
It is not possible to overload a function solely based on its return type. Overloading requires that two functions have either different parameter types, or different numbers of parameters. It is not enough to just have a different return type, as the compiler would not be able to distinguish between the two functions.
Author Profile
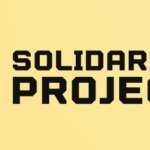
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?