How to Coerce LHS to a List for SEO Optimization
Coercing LHS to a list means forcing the left-hand side of an expression (usually a variable) into a list data type.
Coercing Lhs To A List
Coercing LHS to a list is a process of converting non-list data types into list-formatted data. This is done for better organization, comparison and manipulation of data in a programming language and aids in debugging code. It can also be used to simplify the transfer of data between functions/subroutines. The coercion process works by taking each component of the non-list variable and collecting them into a set of rules applied to the entire set. This results in an ordered list which can then be readily accessed and manipulated within the programming code. In addition, coercing LHS to a Lists allows users to perform certain operations on lists that are otherwise incompatible with a non-list format. Coercing LHS is an invaluable tool for programmers as it provides flexibility, precision, and accuracy in their written code.
Coercing Lhs To A List
Coercing LHS to a list is a process that involves transforming the left hand side of an equation into an ordered set of values or objects. This process is most commonly used when entering data or performing a mathematical operation. It can be used to simplify or clarify data, streamline operations, and reduce errors.
Types of Coercion
Coercion can be classified into two broad types: explicit coercion and implicit coercion. Explicit coercion is when the user explicitly defines the transformation that needs to take place, such as adding a number to each value in a list; while implicit coercion is when the transformation occurs automatically based on the context of the operation.
Benefits of Coercion
The primary benefit of using coercion is that it allows for more efficient data entry and manipulation. By transforming the left hand side into an ordered set, it simplifies operations and reduces errors by eliminating unnecessary steps in entering or manipulating data. Additionally, it can help improve code readability and reduce code complexity, making it easier to debug and maintain code over time. Furthermore, using coercion can also help optimize computational efficiency by reducing time spent on redundant calculations or operations.
Pros & Cons of Coercing Lhs To A List
The advantages of coercing LHS to a list include improved readability and optimization of computational efficiency. Additionally, it can simplify data entry operations and reduce errors due to its ability to transform left-hand side equations into an ordered set. However, there are some potential drawbacks associated with coercing LHS to a list which include increased complexity in debugging code due to its reliance on context-dependent transformations as well as potential issues with maintaining compatibility between different systems when transferring data between them.
Reasons for Coercing Lhs To A List
There are several use cases where coercing LHS to a list can be beneficial such as entering numerical data into spreadsheets or databases, performing mathematical operations in programming languages, or combining multiple datasets for analysis purposes. Additionally, this technique can be useful for simplifying complex equations or visualizing relationships between different sets of values within datasets.
Common Issues with Coercing Lhs To A List
When dealing with coercion issues there are several common issues that can arise such as incorrect transformation due to incorrect context definition or incompatibility issues between different systems when transferring data between them. Troubleshooting these problems often requires careful examination of the code written so far as well as testing different approaches prior to implementation in order to ensure accuracy and compatibility throughout all stages of development.
Different Approaches To Coercing Lhs To A List
There are several optimization ideas that developers can use in order to improve their code’s performance while utilizing coercive techniques such as caching values for reuse during computations instead of performing redundant calculations every time they need to access them. Additionally, alternative solutions such as using built-in functions like map() instead of manually coercing values may provide better performance if implemented properly given certain conditions being met within your project’s environment.
Code Examples of Coercing Lhs To A List
Coercing Lhs To A List enables developers to convert data from one type to another. This process is often used when data needs to be transferred from one system to another, or when dealing with multiple data formats. There are several ways to go about coercing LHS to a list, depending on the programmer’s experience and the complexity of the task.
For short examples, a simple for loop can be used. This loop iterates through each element of the source array and adds it to an array of the desired type. The code might look something like this:
for item in source_array:
list_array.append(item)
More advanced techniques can also be used in order to increase efficiency or accuracy. For instance, a generator expression might be used instead of a for loop, which will reduce memory usage by only creating elements as needed. Additionally, map() can be employed in order to apply functions or transformations across an entire array:
list_array = list(map(function_or_transformation, source_array))
Performance Considerations for Coercing Lhs To A List
When coercing LHS to a list, performance is an important factor to consider. Several techniques can help optimize execution time and avoid unnecessary memory usage. Firstly, it’s best practice to use built-in methods over custom code whenever possible; these are generally more efficient as they have been tested extensively and optimized for speed and memory usage by experts in the language’s development team.
Tuning parameters is also important when considering performance; for example, if using a library function that takes optional arguments such as maximum size or sorting order, it’s best practice to ensure those parameters are set correctly according to the application needs and that they are not left at their default values unnecessarily. Additionally, caching results where possible can help improve performance significantly; this ensures that expensive operations don’t need repeated unnecessarily if the same result is expected multiple times within a single program run.
Ways To Automate Processes With Coercing Lhs To A List
Automating processes with coercing LHS to a list can save time and effort when dealing with large amounts of data or repeating tasks over multiple datasets. There are several tools and libraries available that allow developers quickly automate processes such as mapping functions over arrays or sorting datasets into different formats without having write custom code every time they need these tasks completed; some popular examples include NumPy (for scientific computing) and Pandas (for data analysis). Additionally, there are several strategies available that allow developers create automated pipelines that apply multiple steps at once; these strategies tend involve using intermediary files or databases between steps rather than manually running each step one after the other within a single program run.
Troubleshooting Errors Related To Coercing Lhs To A List
Debugging issues related to coercing LHS to a list can sometimes be difficult due to their complexity; however, there are several strategies available that can help identify errors quickly and accurately without extensive trial-and-error debugging sessions. Firstly, it’s important understand exactly what operations will be performed on each element within an array before attempting any kind of transformation – this helps ensure no unexpected corner cases arise during execution due largely wrong assumptions being made about certain elements’ types or values beforehand. Additionally, it’s best practice log errors whenever possible so that any unexpected behaviour can easily identified later during debugging sessions; this could involve simply printing out relevant information at certain points during execution or writing errors directly into log files which could then be examined later on if necessary. Finally, solving puzzles by breaking them down into smaller steps is often helpful when debugging complex operations such as coercing LHS arrays – this allows developers identify exactly where errors occur more quickly without having spend too much time retracing their steps within long programs or pipelines of code
FAQ & Answers
Q: What is Coercing Lhs To A List?
A: Coercing Lhs To A List is a process of converting an expression on the left-hand side (lhs) into a list of terms. This is often used to simplify complex expressions and enable more efficient calculations.
Q: What are the Types of Coercion?
A: There are two main types of coercion – implicit and explicit coercion. Implicit coercion occurs when the type of data is automatically converted by the compiler, while explicit coercion requires that the programmer explicitly define the type conversion.
Q: What are the Benefits of Coercing Lhs To A List?
A: Coercing lhs to a list can be beneficial for simplifying complex expressions and reducing calculation time. It can also help to reduce errors by ensuring that all types of data are correctly converted before being used in calculations.
Q: What are Common Issues with Coercing Lhs To A List?
A: Common issues with coercing lhs to a list include incorrect conversions, unexpected results, and syntax errors. Troubleshooting these issues requires careful debugging and understanding of the data types being used.
Q: What Different Approaches Can Be Taken Towards Coercing Lhs To A List?
A: Different approaches towards coercing lhs to a list include optimization techniques, alternative solutions, code examples, and automation strategies. Careful consideration should be given to each approach in order to ensure optimal performance.
In conclusion, coercing LHS to a list is a useful technique that can be utilized when a list is required for a function or operation but the existing data is not in the form of a list. It helps to save time by avoiding the need to manually create a list from existing data. This technique is especially helpful when dealing with large datasets, as it allows for faster and more efficient manipulation of data.
Author Profile
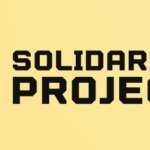
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?