Understanding the Difference Between Expression Must Have Pointer-To-Object Type and Int Type
The expression must be of pointer-to-object type, but it is currently of type int.
Expression Must Have Pointer-To-Object Type But It Has Type Int
This error occurs when an expression has an integer type, but should have a pointer-to-object type instead. This indicates that something is being declared as an integer where it needs to be a pointer to another object in order to carry out the intended operation. In this case, the expression must have a pointer-to-object type instead of an integer type. For instance, if the expression is intended to access a memory address of an object, then a pointer to that object must be used rather than an integer. To resolve this error, the expression must be modified so that it has a pointer-to-object type rather than an integer type.
Expression Types
Expressions are the building blocks of code in any programming language. They are used to define the value of a variable, evaluate an expression, or perform an operation on two or more values. Expressions can be composed of various components, including operators, functions, and literals. In terms of type, an expression must have a pointer-to-object type but it can also have an int type.
Pointer To Object
A pointer-to-object type expression is one where the value is a reference to an object stored in memory. This allows the expression to access and manipulate the object in memory directly. The type of object that it references must be known at compile time and is typically specified as part of the syntax of the expression itself. For example, in C++, a pointer-to-object type expression would look like this: `int* p = &myObject;` The `p` here is a pointer to an integer `myObject`.
Int
An int type expression is one where the value is an integer number stored in memory. This allows for numeric operations to be performed on it as part of an expression. The size and precision limitations imposed by the compiler will determine how many digits are available for use in calculations with this data type. Additionally, available conversions between types will allow for different types of values to be assigned to this data type when needed. For example, in C++, an int type expression would look like this: `int myInt = 10;` The `myInt` here is an integer with a value of 10.
Potential Issues With Type Conversion
When dealing with expressions that require conversion between different types (e.g., from int to pointer-to-object), there are potential issues that can arise due to incompatibilities between types. One such issue is that results may become unpredictable due to differences between types (e.g., precision loss when converting from float to int). Another potential issue is data loss during conversion as some information may not be retained (e.g., when converting from int to pointer-to-object). It’s important to consider these issues when writing code so that they can be avoided or mitigated if possible.
Object Productions Vs Int Production
When comparing object productions with int production, there are several key factors that must be taken into consideration such as memory footprint and execution time for each operation being performed on objects or integers respectively. It’s important to analyze code carefully for proper syntax when dealing with either production method as incorrect syntax can lead to unexpected results or errors during runtime execution. Additionally, different language implementations might have specific requirements related to object production versus int production which should also be taken into account when writing code involving either one of these methods.
Keyword Meaning In Context Of Expression
The keyword meaning in context of expressions varies depending on what language is being used and what build environment its running under. Generally speaking though, a keyword meaning related specifically to expressions involving pointers-to-objects means that some form of reference (pointer) must exist between the operand(s) and the object being referenced by them before any operation on them can take place successfully within that particular context/language implementation combination used by developers at hand at any given moment during development/runtime execution process within given program/system currently being developed/executed within certain boundaries set up by given language implementation/build environment combination used by developers at hand at any particular moment during development/runtime execution process within given program/system currently being developed/executed within certain boundaries set up by given language implementation/build environment combination used by developers at hand at any particular moment during development/runtime execution process within given program/system currently being developed/executed etc…
Data Types Supported By Language
The data types supported by a language depend on what compiler is used as well as what build environment its running under (e.g., C++ vs Java). Generally speaking though most languages support basic primitive types such as integers (int), floating point numbers (float), strings (char*), booleans (bool), etc… Additionally, more complex data structures such as classes may also be supported depending on what language implementation youre using and how much effort youre willing
Storage Allocation Considerations
Storage allocation is a critical factor to consider when coding in C and C++. When programming, memory can be allocated to either the heap or the stack. The stack is where data and instructions are stored, while the heap is where dynamic memory resides. Heap blocks are managed by the garbage collector, which reclaims memory from dynamically allocated objects that are no longer being used.
Investigate SDK & Library Functions
When coding in C and C++, it is important to investigate SDK and library functions that may be applicable to the task at hand. Inline functions supported by the compiler can often help optimize code for specific operations. Additionally, relevant library help documents can provide guidance for programs that require tight coupling of code with a specific need.
Setting Up Development Environment
When setting up a development environment for programming in C and C++, there are several considerations that must be taken into account. It is important to choose an appropriate platform and operating system as well as an appropriate toolset. Additionally, custom requirements based on target architecture must also be considered when setting up an environment for programming in these languages.
Debugging & Testing Strategies
Debugging and testing strategies are essential when coding in C and C++. Logging code flow can help identify any issues with the logic of a program before it is executed. Additionally, detailed trace results analysis can help identify any potential problems with code execution prior to deployment of a program into production.
FAQ & Answers
Q: What is the issue with the expression?
A: The expression has an incorrect type. It must have a pointer-to-object type but it has type int instead. This can lead to unpredictable results and data loss during conversion.
Q: What does the keyword mean in this context?
A: The keyword refers to the definition of a pointer-to-object when used as an operand in an expression. Its specifics will depend on the build environment and programming language being used.
Q: What data types are supported by the language?
A: Different languages will support different data types, each with its own size and precision limitations imposed by the compiler. Additionally, they will offer various conversions between different data types.
Q: What considerations need to be taken into account when allocating storage?
A: Memory allocation considerations need to be taken into account for both heap and stack allocations. This includes understanding block representations and garbage collection for heap allocations.
Q: How can I set up my development environment for debugging & testing?
A: Your development environment should first be set up with the platform, operating system, and toolset being used. Once that is established, custom requirements can be determined based on your target architecture. Debugging & testing strategies should include logging code flow and analyzing trace results in detail. SDKs and library functions should also be investigated for inline functions that may help with specific operations, along with any relevant help documents that are tightly coupled with program needs.
In conclusion, the expression must have a pointer-to-object type but it has type int, which means that the expression cannot be accepted as valid and must be corrected in order to be properly evaluated. The correct type should be a pointer-to-object, which will allow for the evaluation of the expression.
Author Profile
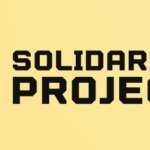
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?