Understanding the Initial Value of Non-Const Reference Must Be an Lvalue
The initial value of a reference to a non-constant must be an object or expression that can be assigned to an lvalue.
Initial Value Of Reference To Non-Const Must Be An Lvalue
Initial Value of References to Non-Const must be an Lvalue addresses the essential role that references play in programming. A reference is a type of object in C++ that provides a way for two objects to refer to one another and/or store data associated with them. This means that when a reference is declared, it has an initial value (usually set at compile time). In order for this reference to be valid, its initial value must be an lvalue, which is an object that can appear on the left-hand side of an assignment operator. If it is not, the program will immediately cause a runtime error. This concept can be confusing to beginners because the syntax of C++ requires lvalues and non-constants (variables) to have different names. It is important for programmers understanding this setup in order to prevent these errors. By having a clear picture of how references work and what conditions they need to fulfill, programmers can write more efficient code with fewer errors.
Definition Of Terms
An lvalue is a type of object or expression which can appear on the left-hand side of an assignment statement. This means that it can be used as the target of an assignment, and it has a memory address to which values can be assigned. A reference is an alias for another object or expression. It refers to the object which it aliases and any operations applied to it are actually applied to its target object.
Meaning Of Non-Const Reference Initialization
Rules of initialization dictate that when initializing a reference, the value must be an lvalue. This means that when declaring a reference variable, it must be initialized with an lvalue or else compilation errors will occur. The most common use case for non-const reference initialization is when creating a reference to an existing variable in order to modify its value without having to pass it around as a parameter.
Benefits Of Lvalue Reference Initialization
The advantage of using lvalue in reference variables is that they provide a more efficient way of accessing values stored in memory than passing them around as parameters. This allows for more concise code and can help improve performance in certain cases. Additionally, when using references with non-const values, developers have more flexibility in terms of what types of data they can manipulate, such as unexpected mutable objects or even dynamic memory locations.
Different Ways To Initialize Non Const References
The standard syntax for initializing non const references involves assigning the address of the value you wish to refer to directly into the reference variable’s declaration statement using the ‘&’ operator followed by the variable’s name. Alternate syntaxes exist on certain platforms which allow for more concise declarations such as ‘ref’ or ‘ref!’.
Problems With Incorrectly Defined Non Const References In Code
When attempting to assign incorrect values into non const references, this can result in unexpected behavior due to narrowing conversions which occur during run time. Narrowing conversions occur when attempting to assign larger data types into smaller containers and may lead to data corruption if not handled carefully.
Impact Of Unintentional Values Assignment Using Narrowing Conversions
When attempting to assign larger data types such as integers into smaller containers such as characters without proper checks in place, this can lead to unexpected behavior due to narrowing conversions taking place during run time which may cause data corruption if not handled properly. For instance, attempting to assign an integer value with more than 8 bits into a single byte character container will lead to unexpected results due its truncation nature during conversion process leading up corrupting stored values within its allocated memory space.
Solutions To Remediate Bugs Caused By Unsafe Conversion
In order avoid bugs caused by unsafe conversion due narrowing conversion process, developers should ensure that all variables being assigned into non const references are validated prior assignment process utilizing language built-in functions such as is_valid() function which ensure that only valid values are assigned prior initialization process; thus mitigating any potential issues related with narrow conversions and ensuring programs safety and soundness at all times without compromising on performance metrics expected from final product output delivered towards end users or consumers alike .Different Programming Guidelines And Best Practice Recommendation To Prevent Reference Variable Errors
It is important for software engineers to develop programming guidelines and best practices that can help prevent errors with reference variables. A few guidelines for avoiding reference-related errors include stack and heap storage management practices, cleaning code by implementing defensive programming tactics, and debugging techniques applied to find issues with declaring and initializing references.
Stack And Heap Storage Management Practices To Be Observed
When developing programs, it is important to consider the proper usage of stack and heap storage management practices. For reference variables, this includes using stack-allocated memory for short-term data preservation and heap-allocated memory for long-term data storage. Furthermore, one should avoid allocating local references on the stack as it often leads to dangling pointers when the function returns. Finally, it is essential to ensure that all allocated memory is properly freed in a timely manner.
Cleaning Code By Implementing Defensive Programming Tactics
In order to produce high quality code, software engineers should strive to implement defensive programming tactics such as using strong type checking and static analysis tools. Additionally, one should use assertions when possible in order to track bugs early on in the development process. Finally, engineers should use unit tests or integration tests whenever possible in order to ensure that all aspects of the program are functioning correctly.
Debugging Techniques Applied To Find Issues With Declaring And Initializing References
In order to efficiently find issues with declaring and initializing references in programs, software engineers should apply various debugging techniques such as trace methods and inspectors. Additionally, common steps for analyzing incorrectly instantiated variables include using breakpoints or logging statements in order to inspect runtime values while an application is running, making use of static analysis tools like valgrind in order to detect memory leaks or other errors related with incorrect initialization of variables.
Consequences Of Implementing Incorrectly Defined Reference Variables In Programs
The consequences of implementing incorrectly defined reference variables can be significant if not taken into account during development processes. These consequences can range from performance degradation due relative to poorly designed data islands or logic boot problems generated due to narrowing conversions. Furthermore, there can be an impact over algorithms consolidation from incorrect implementation of references which may lead to increased overhead factors generated in tight loops or other allocation algorithms used within a program’s source code.
FAQ & Answers
Q: What is an lvalue?
A: An lvalue is a value that identifies an object that persists beyond a single expression. It refers to an object or function that has a memory address and can be used to reference data.
Q: What is the meaning of non-const reference initialization?
A: Non-const reference initialization refers to the process of declaring and assigning values to a non-constant reference variable. This follows certain rules and restrictions, which must be followed for successful initialization.
Q: What are the benefits of using lvalue in reference variables?
A: By using lvalue in reference variables, it allows for easier access to the object in memory as it allows for direct references between two objects. In addition, this also helps reduce code complexity and allows for efficient memory management.
Q: What are the different ways to initialize non const references?
A: Non const references can be initialized using standard syntax or alternate syntax on certain platforms. The standard syntax involves declaring the type of the non const reference variable, followed by an equal sign and then assigning it with an appropriate lvalue. The alternate syntax involves declaring the type of variable followed by parentheses with the appropriate lvalue passed inside.
Q: What are some programming guidelines and best practice recommendations to prevent errors with reference variables?
A: When creating or instantiating a non const reference variable, it should always be done so with an appropriate lvalue, otherwise this could lead to unintended values being assigned as well as narrowing conversions taking place which can cause logic bugs in your program. Additionally, stack and heap storage management practices should be applied as well as defensive programming tactics such as input validation when handling user input to prevent any potential errors when creating or initializing variables.
In conclusion, initializing a reference to a non-constant requires an lvalue, which is a value that can appear on the left-hand side of an assignment. A reference is essentially an alias for an existing object, so the referenced object must already exist in memory and be accessible from the point of declaration. It is important to ensure that the reference is initialized with an appropriate lvalue to avoid errors.
Author Profile
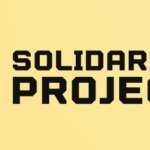
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?