How to Fix Invalid Attempt To Spread Non-Iterable Instance Error
The attempted operation tried to spread an object that is not iterable.
Invalid Attempt To Spread Non-Iterable Instance
In programming, an ‘Invalid Attempt To Spread Non-Iterable Instance’ error occurs when a non-iterable data structure is passed as an argument to the spread operator, such as an Object or a simple value like a number. The spread operator spreads the elements inside of an array or a string into different arguments within a function call, but only works with iterable types like arrays and strings. When these types of non-iterable types are passed instead, the error is thrown and must be corrected in order to proceed with executing the code.
What Is ‘Invalid Attempt To Spread Non-Iterable Instance’?
An ‘Invalid Attempt To Spread Non-Iterable Instance’ error is a type of run-time error that occurs when an attempt is made to spread or iterate over an instance that cannot be iterated. This type of error often occurs when trying to execute a loop or array operation on an object that is not iterable. It is typically due to a misunderstanding of the data types involved in the operation, and can lead to unexpected results.
Understanding The Error Message
Interpreting the message can be challenging as it can appear cryptic and indicate something different than what actually caused the issue. The most important part of resolving this error message is understanding what it means and why it is occurring. In general, this error message indicates that an attempt was made to spread a non-iterable instance, such as an object, string, number, or boolean value.
Common Misunderstandings
When attempting to spread or loop over a non-iterable instance, some developers may mistakenly think that it can be done because they have seen similar actions performed on other data types in their code. However, this type of action cannot be performed on all data types as some are not compatible with looping operations. As such, attempting to do so will result in this error message being thrown.
Steps To Resolve It
Troubleshooting tips for resolving this issue involve first identifying which data type(s) are being used in the loop operation and then determining which ones will support spread operations. Once the correct data types have been identified, they should then be used instead of attempting to use unsupported ones where applicable. Additionally, updated versions of any libraries being used should also be considered as they may contain new features or bug fixes related to this type of issue.
Suggested solutions for resolving this error include refactoring code so that only supported data types are used in loop operations and ensuring that any libraries used are up-to-date with their latest version. Additionally, developers should check their code for any typos or incorrect syntax which could also result in this type of issue occurring.
How Does It Impact Data Processing?
Performance impacts and issues related to invalid attempts at spreading non-iterable instances can range from minor errors being thrown during runtime up to more serious issues such as crashing applications due to incorrect data being processed by loops and arrays. Additionally, these issues can cause delays in processing time due to having to debug and resolve any errors caused by attempting unsupported operations on non-iterable instances.
Common pitfalls and errors related to invalid attempts at spreading non-iterable instances include forgetting which data types support these operations as well as typos or incorrect syntax resulting in unexpected outcomes during runtime execution. Furthermore, failing to update libraries or other dependencies used within code can also lead to unexpected results during runtime execution due these libraries not containing the latest features or bug fixes related to these types of errors.
Defining Iterables and Non-Iterables In ECMAScript & JavaScript
Classification of Variables: When working with ECMAScript & JavaScript variables there are two main categories – Iterables & Non-Iterables – which determine whether or not they can be looped over using certain methods such as map() & forEach(). Iterables are objects which contain multiple values (elements) while Non-Iterables contain only one element (value). Examples include arrays (Iterable) vs numbers/booleans (Non-Iterable).
Data Types Involved: When attempting loop operations on variables it is important to understand the different data types involved as some will support spread operations while others will not; generally speaking strings & objects are Iterables while numbers/booleans/null/ values are Non-Iterables – though exceptions may occur depending on specific implementations used within codebase(s).
Difference Between Array Spread Operator (ES6) & Iteration (ES5)
When it comes to the comparison of array spread operator (ES6) and iteration (ES5), it is important to consider the syntax and methods used for each. The spread operator of ES6 allows a user to unpack an array or object into its individual elements when creating a new array, while iteration in ES5 requires the use of a looping construct for performing operations on each element. With the spread operator, you can simply pass in an iterable object such as an array or object into a function call or assign the elements of an array to variables. This makes it easier and less time consuming than looping through an array using iteration.
Compatibility Considerations
When considering compatibility between different languages, it is important to check language versions that are supported by different implementations. For example, when using ES6 features such as the spread operator, you need to make sure that your version of JavaScript is compatible with the version of JavaScript being used by other applications. Additionally, you should also consider any browser compatibility issues that may arise from implementing new features in older browsers.
Working With Other Languages
When working with other languages, it is important to check language versions and compare any features that are similar between different languages. For example, when working with Python, you can use list comprehension in place of looping through an iterable object. In Java, there is also a similar feature called stream API which allows for operations on collections without having to use loops. Comparing these alternatives can help determine which method would be best suited for the task at hand.
Error Prevention Techniques
In order to prevent errors when implementing new features such as the Array Spread Operator (ES6), it is important to anticipate potential problems and apply practical solutions accordingly. For example, if you are attempting to spread a non-iterable instance such as a string or number then this will result in an error as these objects cannot be split apart into individual elements like arrays can. To prevent this type of error from occurring in your code it is necessary to check whether any variables passed into functions are iterable objects before attempting to spread them out with the operator.
Debugging Techniques
Debugging techniques for invalid attempts at spreading non-iterable instances can vary depending on how they were identified initially and what type of code was being executed at the time of occurrence. When debugging code related issues, interpreted techniques such as stepping through lines sequentially can help identify problems more accurately than automated tools alone which may not always provide sufficient context about where an issue has occurred within code execution flow. Additionally, introducing automated tools such as linting libraries can help catch potential errors before they occur and save time debugging issues that could have been prevented upfront with proper testing protocols in place.
FAQ & Answers
Q: What Is Invalid Attempt To Spread Non-Iterable Instance?
A: Invalid Attempt To Spread Non-Iterable Instance is an error message generated by JavaScript when an attempt is made to spread (using the spread operator) a non-iterable instance. This error is typically seen in ECMAScript or JavaScript code.
Q: What Causes It?
A: This error typically occurs when attempting to perform a spread operation on non-iterable data. This could be caused by attempting to spread a value that is not an array, object, or string. It can also occur if the data type of the value being operated on is not supported by the language being used.
Q: How Can I Interpret The Error Message?
A: The Invalid Attempt To Spread Non-Iterable Instance error message is fairly self-explanatory and can be interpreted as an indication that the code attempted to spread a non-iterable instance. In other words, the code attempted to operate on data that cannot be iterated over using standard methods such as for loops or map functions.
Q: What Steps Can I Take To Resolve It?
A: If you are encountering this error, there are several steps you can take to try and resolve it. These include troubleshooting why your code attempted to spread a non-iterable instance in the first place, suggesting solutions for how your code could be modified to prevent this from occurring in future iterations, and introducing automated tools to help debug any potential issues with your code.
Q: How Does It Impact Data Processing?
A: When an Invalid Attempt To Spread Non-Iterable Instance error occurs during data processing operations, it can cause significant performance impacts and errors in your program’s output. Common pitfalls include attempting to use incompatible data types within a loop or using unsupported methods such as attempting to use array spread operators with ECMAScript 5 syntax instead of ES6 syntax.
The conclusion to the question ‘Invalid Attempt To Spread Non-Iterable Instance’ is that this is an error caused when trying to spread an object which is not iterable. This means that the object does not have a defined “iteration protocol” which allows it to be iterated over. The error can be resolved by ensuring that the object being spread is in fact an iterable object.
Author Profile
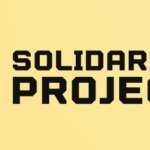
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?