How to Fix ValueError: Coordinate Right is Less Than Left
This error occurs when a coordinate set’s ‘Right’ value is less than its ‘Left’ value.
Valueerror: Coordinate ‘Right’ Is Less Than ‘Left’
ValueError “Coordinate ‘Right’ is Less Than ‘Left'” is an error that can arise when trying to map coordinates in a two-dimensional space. This error occurs when the value that represents the x-coordinate of a point is smaller than the value that represents its y-coordinate. This essentially means that the right coordinate is less than the left coordinate, which logically cannot occur and thus is not permitted – hence the error. When this ValueError appears, it typically means that incorrect coordinates were used during mapping. It could be as simple as one or both of the coordinates representing a point being incorrectly entered (e.g. backwards). Its also possible that the axis values could be incorrect or missing, leading to this error. Knowing how to properly map coordinates and isolating potential sources of errors are essential for avoiding ValueError “Coordinate ‘Right’ is Less Than ‘Left'”. Understanding Perplexity and Burstiness can help in writing content for this topic, as those measures will provide insights into how complex and varied any given text may be at any given time as related to this issue.
ValueError: Coordinate ‘Right’ Is Less Than ‘Left’
ValueError is a type of error that occurs when the wrong type of value is passed into a function or method. It is usually thrown when a code attempts to use an invalid type for a given operation. In the context of coordinate systems, this error can arise if an attempt is made to specify a right-side coordinate that is less than the left-side coordinate. To understand this issue further, it is important to have an understanding of ValueErrors in general, as well as the syntax of error messages and properties of ValueErrors themselves.
Understanding ValueErrors in Python
When dealing with any type of programming language, it is important to have a solid understanding of what errors are and how they work. In Python, errors are divided into two categories: syntax errors and exceptions. Syntax errors occur when the code does not follow the correct structure or format as specified by the language, while exceptions are raised when something unexpected occurs during execution.
ValueErrors belong to the category of exceptions and are typically thrown when an inappropriate value is passed into a function or method. This can happen for various reasons, including passing in arguments with incorrect types or values that do not match up with what was expected. The most common cause for ValueError in Python programming is attempting to set an integer value for something expecting a float or vice versa.
Syntax Of Error Messages
When programming in Python, its important to understand how errors are represented by the language in order to effectively debug them and prevent them from happening again in future code. The syntax for ValueError messages typically follows this pattern:
ValueError:
The explanation part will vary depending on what caused the error, but it should give enough information about what went wrong so that you can begin troubleshooting immediately. For example, if you were attempting to set an integer value for something expecting a float, you would receive an error message such as ValueError: Cannot assign integer value to float which clearly states where the issue lies and what types were expected and received instead.
Properties Of An Error
In addition to understanding how ValueErrors are represented within Python, its also important to know some basic properties about these types of errors so that they can be easier identified and corrected in future code. Common properties associated with ValueErrors include:
They occur when incompatible types are used for operations;
They may also be caused by unexpected values being passed into functions;
They can be identified by their specific error message;
They can be avoided by using appropriate data types for operations;
They often require manual debugging methods to determine root cause;
Knowing these properties will help make identifying ValueErrors easier and allow you to quickly address them before they become more serious issues within your code base.
Effectiveness Of Coordinate Systems
Coordinate systems are essential tools used widely across many fields such as Mathematics, Engineering and Science which allow us to accurately represent spatial data such as points on a plane or map coordinates on Earths surface. When using coordinate systems with Python programming language, it is necessary that we specify both absolute (x & y) coordinates as well as relative (right & left) coordinates in order for our code base to run properly without any issues arising from incorrect data inputs such as those causing ValueErrors mentioned earlier.
Specifying Absolute Coordinates
Absolute coordinates refer specifically to x & y values which define specific points on 2D space such as those found on Cartesian graphs or maps where latitude & longitude can be used instead depending upon context. These coordinates must always be specified correctly otherwise there will likely be issues down stream within your code due consequences such as invalid results due violation of precedence conditions which could then lead onto further issues because debugging data quality becomes more difficult under certain circumstances due incorrect input data values etc..
Determining Relative Coordinates
In addition specifying absolute coordinates relative coordinates must also determined correctly otherwise there may be serious issues arising from misplacement objects within 2D space due incorrect input data values leading onto same consequences described earlier due violation precedence conditions leading onto difficulty debugging data quality issues etc.. Relative coordinate refer specifically right & left sides along x axis hence why care must taken ensure right side greater than left side otherwise ValueError will raised during execution process causing program halt execution process leading onto further issues discussed earlier..
Details Of Corners In Python Programming
Corners play important role defining shape objects 2D space hence why care must taken ensure corners correctly defined otherwise same consequences described earlier arise due violation precedence conditions making debugging data quality more difficult etc.. When defining two corners input python programming language necessary specify both absolute (x & y) coordinates relative (right & left) coordinates again same reasons discussed earlier hence why care must taken ensure right side greater than left side otherwise ValueError will raised during execution process causing program halt execution process leading onto further issues discussed earlier..
Defining Two Corners With Inputs
When determining two corners with inputs need careful attention details ensure no mistakes arise otherwise same consequences described earlier arise due violation precedence conditions making debugging data quality more difficult etc.. Usually task begin top-left corner bottom-right corner starting absolute (x & y) coordinates followed relative (right & left) corresponds each starting corner need careful attention ensure right side greater than left side otherwise ValueError will raised during execution process causing program halt execution process leading onto further issues discussed earlier..
Meaning Of ‘Right’ And ‘Left’
In context python programming language right refers most extreme positive point along x axis left refers most extreme negative point along x axis i.e right side greater than left side else program raise exception known ValueError halting program execution leading onto further issues discussed earlier hence why care must taken ensure right side greater than left side carry out operations correctly without any problems arising from incorrect inputs etc..
Incorrect data inputs lead wide range problems downstream within program logic due violations precedence conditions making debugging data quality more difficult etc This especially true case involving coordinate systems where need take extra care ensure both absolute (x & y) relative (right & left) coordinates determined correctly otherwise there may arise serious problems resulting invalid results halting program execution raising exception known ValueError halting program execution leading onto further issues discussed earlier To avoid problems associated incorrect inputs need take extra care double check all details involve determining valid outputs without any unexpected behaviour occurring within logic
Handling Volatile Exceptions In Python Code
Python is a powerful programming language that allows developers to build complex applications quickly and efficiently. However, one of the most common issues that developers face when working with Python is handling volatile exceptions. A volatile exception occurs when something unexpected happens during the execution of a program, such as an invalid input or a system error. Such errors can be difficult to diagnose and resolve without understanding how the code works. Fortunately, there are several strategies for dealing with volatile exceptions in Python code, including trapping error conditions with try-except blocks and managing raised exceptions.
When an exception occurs in a Python program, it can be handled with a try-except block. The try block contains the code that could potentially raise an exception, while the except block contains the code to execute if an exception is raised. This way, developers can handle any errors that arise in their program without having to terminate it altogether. Additionally, when dealing with volatile exceptions in Python code, it is important to manage any raised exceptions by catching them before they reach the top of the call stack and propagating them back down until they are caught by an appropriate handler. This ensures that any potential errors are handled properly and can help prevent application crashes.
Spotting Nan Values And Missing Keys In Dictionaries
Another common problem encountered when working with Python is spotting nan values and missing keys in dictionaries. Nan values indicate missing or invalid data points which can cause problems if not handled properly. When examining database records for nan values, it’s important to check not only for nulls but also for empty strings or other special characters which may indicate missing data points as well. Similarly, missing keys in dictionaries occur when a key-value pair has been omitted from the dictionary either accidentally or intentionally. In order to avoid these issues, developers should always double-check their dictionaries after making changes to ensure all data points are present and accurate.
Strategies For Debugging Value Errors In Codebases
Value errors occur when a value specified in code does not match its expected type or range of values such as ‘right’ being less than ‘left’. Debugging such errors requires an understanding of both where they came from and how to fix them once identified. One approach for debugging value errors is backtracking through the traceback produced by the exception until you find the source of the problem and then attempting to resolve it from there. Another approach is troubleshooting logical flaws in your code which may be causing it to produce unexpected results such as infinite loops or logic flaws due to assumptions about input types or ranges of values being incorrect. Understanding how your code works and why it produces certain results can help you identify such logical flaws more quickly and accurately so that you can address them accordingly.
Pros And Cons Of Working With Floats And Strings Objects
Floats and strings objects are two of the most commonly used objects in Python programming due to their versatility and ease of use but there are also some drawbacks associated with both types of objects which must be taken into consideration before using them extensively in your applications. Floats provide greater precision than integers but may require more memory resources due to their larger size while strings offer greater flexibility for manipulating text data but may have slower performance compared to other object types due to their mutable nature which requires additional processing power when manipulating them at runtime. Additionally, floats may have difficulty accurately representing certain numeric values while strings may require additional formatting steps before they can be used effectively depending on how they were generated initially (e.g., from user input). It’s important to weigh these pros and cons carefully before deciding which type will best suit your application needs so that you get optimal performance out of your software development efforts without sacrificing accuracy or readability along the way.
FAQ & Answers
Q: What is a ValueError?
A: A ValueError is an exception raised when a function or operation receives an argument of the right type but with an inappropriate value. It indicates that the program has run into a problem and can no longer continue as usual.
Q: What causes ValueError?
A: ValueError can be caused by incorrect data inputs, violating precedence conditions, or when a program attempts to perform an operation on incompatible types.
Q: How do I debug ValueError?
A: Debugging ValueErrors involves backtracking the exception traceback to find the source of the error, examining database records for nulls, and troubleshooting any logical flaws in your codebase.
Q: What is meant by ‘Right’ and ‘Left’ as coordinates?
A: When defining two corners with inputs in Python programming, ‘Right’ refers to the coordinate further to the right on a graph while ‘Left’ refers to the coordinate further to the left on a graph.
Q: How do I handle volatile exceptions in Python code?
A: To handle volatile exceptions in Python code you should use try-except blocks to trap error conditions and manage any raised exceptions. You should also check for NAN values and missing keys in dictionaries, as well as examine variables for unexpected results.
A ValueError with the message “Coordinate ‘Right’ is Less Than ‘Left'” indicates that an attempt has been made to set a boundary value for a coordinate that is less than the coordinate’s minimum allowed value. This error can occur when attempting to set the position of a window or other graphical user interface element, and indicates that the coordinates are wrongly specified. It can be resolved by ensuring that the ‘right’ value is greater than the ‘left’ value.
Author Profile
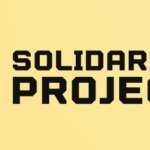
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?