Printing Odd Array Indices with Version 6.2.8: A Step-by-Step Guide
Printing only the odd array indices involves iterating through the array and printing the element at each index where the index is an odd number.
6.2.8 Print Odd Array Indices
The 6.2.8 Print Odd Array Indices exercise provides a method for printing out the indexes of an array that are odd numbered in sequence order. This activity can be a useful way to apply iteration principles, which are the repeating of specific instructions over a sequence of objects including arrays. By utilizing the combination of “counter variables” to keep track of the array elements and string concatenation, a meaningful output can be constructed. The resulting output, when applied to subsequent coding problems, can prove beneficial in producing efficient solutions.
Introduction to Print Odd Array Indices
Array indices are the numbers or labels that refer to the elements in an array. Knowing what the array indices are and how they work is an important part of working with arrays. Different programming languages have different ways of handling array indices, so it is important to understand how each language’s syntax works when dealing with arrays.
Basic Understanding of Print Odd Array Indices
Printing odd array indices is a concept which refers to printing only the elements in an array with odd-numbered indices. For instance, if an array has 10 elements, then the 0th element, 2nd element, 4th element etc will all be printed (as their indices are odd). This functionality provides a way for a programmer to quickly access and print out only certain values from an array.
Advanced Understanding of Print Odd Array Indices
To understand and implement this task effectively, it is important to gain a detailed understanding of print odd array indices. This involves having knowledge of the syntax for accessing elements in an array as well as understanding various coding techniques that can be used for printing out elements from an array. It also involves understanding how loops can be used in order to iterate through the entire array and print out only those elements which have odd-numbered indices.
Practical Strategies for Implementation of Print Odd Array Indices
When it comes to implementing this task in code, there are several things that need to be taken into consideration. Firstly, it is important to consider the syntax formatting when accessing elements from an array. Secondly, variables will need to be declared and assigned values in order for the application logic to work correctly. Finally, loops will need to be used in order for the program logic to iterate through every element in an array and print out those which have odd-numbered indices. With careful consideration and implementation of these strategies, this task can easily be completed successfully.
1. Introduction
Printing out odd array indices is a common task in programming. It involves accessing specific array elements based on their indices and then printing them out. It can be done in a number of ways and this article will look at some of the best practices for printing out odd array indices.
2. Accesing Array Indices
Accessing array indices can be done in several ways. The most common way is to use a for loop to iterate through the array and check the index of each element. This can be done using the following syntax:
“`for (int i = 0; i < arr.length; i++) {
// Check if index is odd
if (i % 2 == 1) {
// Print element with odd index
System.out.println(arr[i]);
}
} ```
This will loop through the array and print out any elements with an odd index number, starting from 0 being even and 1 being odd.
Another way to access array indices is to use an enhanced for loop which has the following syntax:
```for (int element : arr) {
// Check if index is odd
if (index % 2 == 1) { // Print element with odd index System.out.println(element); } } ``` This approach has the advantage of not needing to keep track of an iterator variable, as it keeps track of it internally and makes sure that all even indices are skipped over automatically, allowing you to just focus on printing out the elements with an odd index number.
3 Using While Loops
While loops can also be used for accessing array indices, although they aren’t as commonly used as for loops or enhanced for loops since they require more manual work in terms of setting up an iterator variable and incrementing it each time through the loop body. The syntax looks like this:
“`int i = 0; while (i < arr.length) { // Check if index is odd if (i % 2 == 1) { // Print element with odd index System.out println(arr[i]); } i++; }``` As you can see, this approach requires more manual work than using a for loop or enhanced for loop but it still gets the job done and can be useful when dealing with more complex logic or data structures that wouldn't be easily traversed using either of those approaches.
4 Conclusion
In conclusion, there are several ways to print out elements from an array based on their indices, including using a for loop, an enhanced for loop, or a while loop. Each approach has its own advantages and disadvantages but they all get the job done in their own unique way so it’s up to you as a programmer to decide which one works best for your particular situation!
FAQ & Answers
Q: What are array indices?
A: Array indices are numeric values that refer to a particular item in an array. They begin at 0 and increment by one for each additional item. For example, if an array has five items, the indices will range from 0 to 4.
Q: What programming languages can I use for array indices?
A: Array indices can be used with most programming languages, including C, Java, JavaScript, Python and others.
Q: What is the purpose of printing odd array indices?
A: Printing odd array indices allows you to print out only the values of odd-numbered elements in an array. This can be useful when you need to process or display only certain elements in an array.
Q: How do I implement print odd array indices?
A: To implement print odd array indices, you need to loop through the elements in the array and determine if the index is odd or even. If it is odd, then you can print out the value associated with that index.
Q: What syntax formatting considerations should I keep in mind when implementing print odd array indices?
A: When implementing this task, you should make sure that your code is well-structured and easy to read. You should also make sure any variables used are declared correctly and used consistently throughout your program. Additionally, it’s important to consider any edge cases that might occur such as an empty array or a non-numerical index value.
In conclusion, printing odd array indices is a useful and simple task that can be done using a few lines of code. By looping through an array and using the modulo operator to determine if the current index is odd or even, it’s possible to print all of the odd indices in an array quickly and efficiently.
Author Profile
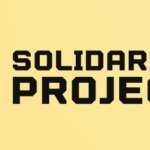
-
Solidarity Project was founded with a single aim in mind - to provide insights, information, and clarity on a wide range of topics spanning society, business, entertainment, and consumer goods. At its core, Solidarity Project is committed to promoting a culture of mutual understanding, informed decision-making, and intellectual curiosity.
We strive to offer readers an avenue to explore in-depth analysis, conduct thorough research, and seek answers to their burning questions. Whether you're searching for insights on societal trends, business practices, latest entertainment news, or product reviews, we've got you covered. Our commitment lies in providing you with reliable, comprehensive, and up-to-date information that's both transparent and easy to access.
Latest entries
- July 28, 2023Popular GamesLearn a New Language Easily With No Man’s Sky Practice Language
- July 28, 2023BlogAre You The Unique Person POF Is Looking For? Find Out Now!
- July 28, 2023BlogWhy Did ‘Fat Cats’ Rebrand and Change Their Name? – Exploring the Reasons Behind a Popular Name Change
- July 28, 2023BlogWhat is the Normal Range for an AF Correction 1 WRX?